Zoom MKMapView to fit polyline points
Solution 1
-(void)zoomToPolyLine: (MKMapView*)map polyline: (MKPolyline*)polyline animated: (BOOL)animated
{
[map setVisibleMapRect:[polyline boundingMapRect] edgePadding:UIEdgeInsetsMake(10.0, 10.0, 10.0, 10.0) animated:animated];
}
Solution 2
-(void)zoomToPolyLine: (MKMapView*)map polyLine: (MKPolyline*)polyLine
animated (BOOL)animated
{
MKPolygon* polygon =
[MKPolygon polygonWithPoints:polyLine.points count:polyLine.pointCount];
[map setRegion:MKCoordinateRegionForMapRect([polygon boundingMapRect])
animated:animated];
}
Solution 3
in swift:
if let first = mapView.overlays.first {
let rect = mapView.overlays.reduce(first.boundingMapRect, combine: {MKMapRectUnion($0, $1.boundingMapRect)})
mapView.setVisibleMapRect(rect, edgePadding: UIEdgeInsets(top: 50.0, left: 50.0, bottom: 50.0, right: 50.0), animated: true)
}
this will zoom/pan to fit all overlays with a little buffer
Solution 4
You could loop through all CLLocations
recording the max/min
coordinates and use that to set a view rect like they do on this question iOS MKMapView zoom to show all markers .
Or you could go through each of your overlays and get their boundingMapRect
then use MKMapRectUnion
(http://developer.apple.com/library/ios/documentation/MapKit/Reference/MapKitFunctionsReference/Reference/reference.html#//apple_ref/c/func/MKMapRectUnion) to combine them all until you have one MKMapRect
that covers them all and use that to set the view.
[mapView setVisibleMapRect:zoomRect animated:YES]
This question shows a simple loop combining the maprects in unions as I suggested: MKMapRect zooms too much
Solution 5
Swift 3 version of garafajon excellent code
if let first = self.mapView.overlays.first {
let rect = self.mapView.overlays.reduce(first.boundingMapRect, {MKMapRectUnion($0, $1.boundingMapRect)})
self.mapView.setVisibleMapRect(rect, edgePadding: UIEdgeInsets(top: 50.0, left: 50.0, bottom: 50.0, right: 50.0), animated: true)
}
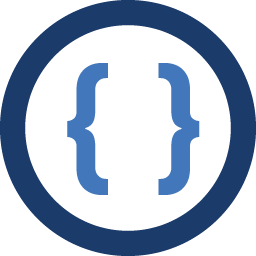
Admin
Updated on June 21, 2022Comments
-
Admin almost 2 years
I have an array, allCollections, that holds programmatically-created arrays of CLLocations the user has recorded through my iOS app. Each sub-array in allCollections holds all the location points in a trip taken.
I draw MKPolylines off of the CLLocations in the arrays in allCollections to represent those trips on an MKMapView. My question is this: With the polylines added to the map, how would I go about programmatically zooming and centering the map to display all of them?