'MyhomePage({Key key, this.title}) : super(key: key);' in Flutter - what would be a clear explanation with an example?
Solution 1
The code is the constructor of the MyHomepage
widget.
{Key key, this.title}
It declares two optional named parameters (optional named because of {}
) where
the first is of name
key
with typeKey
the second is of name
title
with the type of the fieldthis.title
and automatically initializesthis.title
with the passed value. This is nice syntactic sugar that saves some writing.
:
starts the initializer list.
The initializer list allows some to execute some expressions before the call is forwarded to the constructor of the super class.
When a class is initialized, read access to this
is forbidden until the call to the super constructor is completed (until the body of the constructor is executed - in your example the constructor has no body).
The initializer list is often use to validate passed parameter values with assert(key != null)
or to initialize final
fields with calculated values (final
fields can't be initialized or updated later).
super(key: key)
forwards to the constructor of the super class and passes the parameter key
passed to MyHomepage
to the super constructors key
parameter (same as for MyHomepage({Key key})
).
Solution 2
Thanks for Günter's detailed explanation which helped me at the very beginning. Here I would like to explain the background for this question, and especially the punctuation as syntax a little bit.
The mentioned line of code,
MyHomepage({Key key, this.title}) : super(key: key);
should come from the auto-generated Flutter application boilerplate.
The complete context is:
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
// This widget is the home page of your application. It is stateful, meaning
// that it has a State object (defined below) that contains fields that affect
// how it looks.
// This class is the configuration for the state. It holds the values (in this
// case the title) provided by the parent (in this case the App widget) and
// used by the build method of the State. Fields in a Widget subclass are
// always marked "final".
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
For me, the weird punctuation (curly brackets{ }
and two colons :
) is what prevented me from comprehending its syntax.
Curly brackets of {Key key, this.title}
: is the syntax for declaring optional parameters while defining function in Dart.
The first colon of MyHomepage(...) : super(key: key)
is a separator that specifies the initializer list (super(key: key)
) of constructor function MyHomepage(...)
The second colon within super(key: key)
is the way how you pass a parameter to a named function (super()
in this case).
- For example, a function
enableFlags
is defined as follows
void enableFlags({bool bold, bool hidden}) {...}
- To call the function, the way Dart passes parameter to the function, is by declaring
parameterName
beforevalue
, separated with colon:
, which is safer for developer than the Pythonic way. The counterpart syntax in Swift should be external parameter.
enableFlags(bold: true, hidden: false);
I wish this could help.
All the definitions and examples can be found in Dart's official document.
Solution 3
Flutter uses Keys for a lot of things inside the framework. Since your widget extends StatelessWidget or StatefulWidget, this is the way of your widget having a key so that the framework knows what to do with it (the super function is passing the key to the superclass widget - which can be understood by the framework).
Except for that is only Dart syntax (which is awesome by the way).
You can learn more about Keys here: https://www.youtube.com/watch?v=kn0EOS-ZiIc
Another example is the testing framework. Flutter driver needs a key to know which widget to look for and interact with it. With this syntax your widget will have a key and it will work!
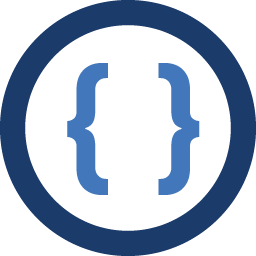
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
In Flutter, what would be a clear explanation with an example?
My confusion is about
key
, as in the code below.MyHomepage({Key key, this.title}) : super(key: key);
-
Sorin over 3 yearsI'm new to Flutter/Dart, but from Dart docs I understood
{Key key, this.title}
is the syntax for declaring named parameters not optional parameters (aldo it's true that named parameters are optional by default). What I'm confused about is the use ofthis.title
in a named parameter declaration. As I understant it, this syntax declares a parameter namedtitle
and also initialises thetitle
property of the object with the value passed for thetitle
parameter. -
Neal.Marlin about 2 years@Sorin I have to say that the latter is really an ugly syntax even though it looks like more brief.