How to store a User id or "key" after login and access from anywhere in Flutter app?
1,616
you can use static and a class to save this data in your entire app
class SaveData{
static int id
setId(int id1){
id = id1;
}
getId(){
return id;
}
}
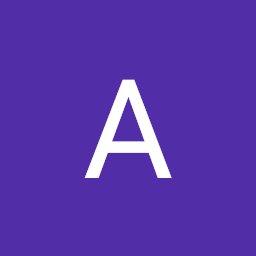
Author by
Anna Smith
Updated on December 28, 2022Comments
-
Anna Smith over 1 year
I am trying to determine the best way to store and access my Flutter app's User object after a login. There is no real authentication as of right now. So when the "user" logs in it just checks a json file for a dummy username and password. The only thing I want to know is what is the appropriate way to store and access my User id from anywhere in the app? I have been passing it as a route parameter every time the screen changes but that seems like overkill. What is the most appropriate way to do this? Just create a globals.dart file and add the user id as a variable?
Example of User in json:
{ "id" : 5, "fName" : "John", "lName" : "Doe", "position" : "Software Developer", "username" : "jdoe", "password" : "jdoepass", "imageUrl": "assets/images/profile_pictures/profilePicture.png", "email" : "[email protected]", "location" : "Big Software Company" }
Login.dart:
import 'package:flutter/material.dart'; import 'package:google_fonts/google_fonts.dart'; import '../utils/opWidgets.dart'; import '../utils/fetchJson.dart'; import '../../entities/User.dart'; /* Description: Login screen widget and functions to handle fetching user json */ class LoginPage extends StatefulWidget { @override State<StatefulWidget> createState() { return _LoginPageState(); } } class _LoginPageState extends State<LoginPage> { List<User> _userList = []; //used to represent the list items in the UI final userTxtController = TextEditingController(); final passTxtController = TextEditingController(); User _currentUser; bool _invalidUserMsgVisible = false; @override initState() { //initializes data before build() is called //Use this method to initialize data that will be displayed //initialize list items using function that fetches/converts json fetchUsers().then((value) { setState(() { _userList.addAll(value); }); }); super.initState(); } @override void dispose() { //releases memory userTxtController.dispose(); passTxtController.dispose(); super.dispose(); } @override Widget build(BuildContext context) { return Scaffold( resizeToAvoidBottomInset: false, body: GestureDetector( //Makes the keyboard collapse when the scaffold body is tapped onTap: () => FocusScope.of(context).unfocus(), child: _layoutDetails(), ), ); } //This widget determines the layout of the widgets _logo() and _loginForm() based on the screen's orientation Widget _layoutDetails() { Orientation orientation = MediaQuery.of(context).orientation; if (orientation == Orientation.portrait) { return Column( mainAxisAlignment: MainAxisAlignment.center, children: [ _logo(), _loginForm(), ], ); } else { return Row( mainAxisAlignment: MainAxisAlignment.center, children: [ _logo(), Container( width: MediaQuery.of(context).size.width / 1.8, height: MediaQuery.of(context).size.height, child: _loginForm(), ), ], ); } } Widget _logo() { return Container( width: 225, child: Image.asset('assets/images/logos/OPNewLogo.png'), ); } Widget _loginForm() { return Container( width: double.infinity, padding: EdgeInsets.all(20), child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Visibility( visible: _invalidUserMsgVisible, child: Text( 'Username or password is invalid', style: GoogleFonts.montserrat(color: Colors.red, fontSize: 14), ), ), SizedBox(height: 5), Container( //margin: EdgeInsets.symmetric(horizontal: 10), width: 500, child: opTextField('Username', false, userTxtController), ), SizedBox(height: 20), Container( //margin: EdgeInsets.symmetric(horizontal: 10), width: 500, child: opTextField('Password', true, passTxtController), ), SizedBox(height: 20), Container( width: 500, height: 40, padding: EdgeInsets.symmetric(horizontal: 8), child: ElevatedButton( onPressed: () => _verifyLogin( userTxtController.text.toString().trim(), passTxtController.text.toString(), ), child: Text( 'LOGIN', style: GoogleFonts.montserrat( fontSize: 16, letterSpacing: 1.5, color: Colors.white, fontWeight: FontWeight.w500, ), ), style: ElevatedButton.styleFrom( shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(20), ), primary: Theme.of(context).accentColor, elevation: 3, ), ), ), SizedBox(height: 5), Container( width: 500, height: 40, padding: EdgeInsets.symmetric(horizontal: 8), child: ElevatedButton( onPressed: () => { Navigator.pop(context), }, child: Text( 'REGISTER', style: TextStyle( fontSize: 16, letterSpacing: 2.2, color: Theme.of(context).shadowColor, fontWeight: FontWeight.w500), ), style: ElevatedButton.styleFrom( shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(20), side: BorderSide( color: Theme.of(context).primaryColorDark, ), ), primary: Colors.white, ), ), ), Container( child: TextButton( autofocus: false, clipBehavior: Clip.none, child: Text( 'Forgot Password', style: GoogleFonts.montserrat( fontSize: 15, color: Theme.of(context).shadowColor, decoration: TextDecoration.underline, ), ), onPressed: () => Navigator.pushNamed(context, resetPasswordPageRoute), ), ), ], ), ); } //Function to check the username and password input against the list of user objects void _verifyLogin(String username, String password) { bool _validUser = false; //Loop through fetched user objects and check for a match for (var user in _userList) { if (username == user.username && password == user.password) { _validUser = true; _currentUser = user; //Set the current user object break; } } //If a valid user was found, pop login screen and push home screen if (_validUser) { Navigator.popAndPushNamed( context, homePageRoute, arguments: _currentUser, //Pass the current user object to the home screen ); } else { //if no valid user was found, make error message text visible setState(() { _invalidUserMsgVisible = true; }); } } }