Flutter Auto Login using local database
I have used SharedPreferences instead of local database and that worked.
Below is the minimal implementation
import 'package:IsBuddy/Screens/Dashboard/dashboard.dart';
import 'package:IsBuddy/Screens/Login/login_screen.dart';
import 'package:flutter/material.dart';
import 'package:shared_preferences/shared_preferences.dart';
class AutoLogin extends StatefulWidget {
@override
_AutoLoginState createState() => _AutoLoginState();
}
class _AutoLoginState extends State<AutoLogin> {
Future<SharedPreferences> _prefs = SharedPreferences.getInstance();
Future<bool> logged;
@override
void initState() {
logged = _prefs.then((SharedPreferences prefs) {
return (prefs.getBool('logged') ?? false);
});
super.initState();
}
@override
Widget build(BuildContext context) {
return FutureBuilder<bool>(
future: logged,
builder: (BuildContext context, AsyncSnapshot<bool> snapshot) {
switch (snapshot.connectionState) {
case ConnectionState.waiting:
return Container(
child: Center(
child: CircularProgressIndicator(),
));
default:
if (snapshot.hasError) {
return Text('Error: ${snapshot.error}');
} else {
return snapshot.data ? Dashboard() : LoginScreen();
}
}
},
);
}
}
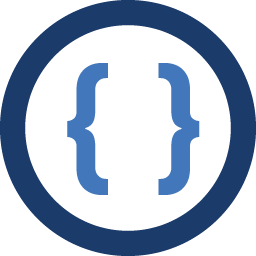
Admin
Updated on December 21, 2022Comments
-
Admin over 1 year
I'm new to flutter and trying to create a login app.
I have 2 screens.
- Login (If user enters correct credentials, store user information to local db(sqflite) and navigate to home).
- Home (have logout option).
I'm trying to achieve auto login i.e when user closes the app without logging out, the app should navigate to home automatically without logging again when app reopens.
My logic: If user enters valid credentials, clear the db table and insert newly entered credentials. Auto login - when app starts, check if record count in db table is 1, then navigate to home else login.
Here's the code which I have tried:
class MyApp extends StatefulWidget { @override _MyAppState createState() => _MyAppState(); } class _MyAppState extends State<MyApp> { final dbHelper = DatabaseHelper.instance; bool logged = false; @override void initState() { super.initState(); autoLogIn(); } void autoLogIn() async { if (await dbHelper.queryRowCount() == 1) { setState(() { logged = true; }); return; } } @override Widget build(BuildContext context) { return logged ? HomeScreen(): LoginScreen(); } }
It makes me as if, the widget is build before the state of logged is changed. How can I achieve auto login assuming there is no issue with database(sqflite) implementation.
-
EraftYps almost 4 yearsInstead of storing the credentials in the database, save a boolean in shared preferences and retrieve it when the app starts and navigate to the desired screen.
-
Admin almost 4 years@EraftYps Yes! That worked. Thanks