404 error when Routing using Express and Nodejs
Two issues here :
(1) Looks like the router.route().all
was not returning a result, or calling the next()
route in the layer.
There is an article here also. https://groups.google.com/forum/#!topic/express-js/zk_KCgCFxLc
If I remove the .all or insert next()
into the .all
function, the routing works correctly.
(2) the trailing'/'
in the route definition was causing another error
i.e. router.route('/users/')
should be router.route('/users')
The slash is important.
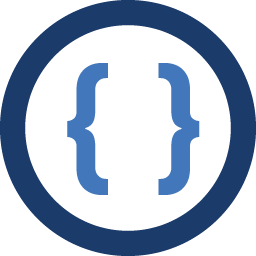
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have created a basic Node/Express App and am trying to implement routes based on separation of logic in different files.
In Server.js
var app = express(); var router = express.Router(); require('./app/routes/users')(router); require('./app/routes/events')(router); require('./app/routes/subscribe')(router); require('./app/routes/login')(router); app.use('/api',router);
In ./app/routes/users.js
module.exports = function(router){ router.route('/users/') .all(function(req, res, next) { // runs for all HTTP verbs first // think of it as route specific middleware! }) .get(function(req, res, next) { res.json(req.user); }) .put(function(req, res, next) { // just an example of maybe updating the user req.user.name = req.params.name; // save user ... etc res.json(req.user); }) .post(function(req, res, next) { next(new Error('not implemented')); }) .delete(function(req, res, next) { next(new Error('not implemented')); }) router.route('/users/:user_id') .all(function(req, res, next) { // runs for all HTTP verbs first // think of it as route specific middleware! }) .get(function(req, res, next) { res.json(req.user); }) .put(function(req, res, next) { // just an example of maybe updating the user req.user.name = req.params.name; // save user ... etc res.json(req.user); }) .post(function(req, res, next) { next(new Error('not implemented')); }) .delete(function(req, res, next) { next(new Error('not implemented')); }) }
All of the routes are returning 404-Not Found.
Does anyone have suggestions on the best way to implement modular routing in Express Apps ?
Is it possible to load multiple routes in a single Instance of express.Router() ?
------------Edit---------------
On Further Testing
I've been able to debug the express.Router() local instance, and the routing layer stack in the local "router" variable is being updated with the routes from the individual modules.
The last line:
app.use('/api', router);
is also successfully updating the global app instance internal app.router object with the correct routing layers from the local router instance passed to it.
I think the issue is that the Routes for the '/api' are at number 13-14 in the routing layer stack so there is some issue further up the stack with some other middleware routing not letting the routes through to the end... I just need to track this down I guess.
-
Admin over 9 yearsI understand that this will work, but I would specifically like to know whether it can be implemented using a single instance of express.Router(). This implementation requires that the base URL is explicitly defined for each route. Using a single instance of express.Router(), I'm hoping to set the base url route to '/api' and add routes on top of that.