Access parent class instance attribute from child class instance?
Solution 1
Parent is a class - blue print not an instance of it, in OOPS to access attributes of an object it requires instance of the same, Here self/child is instance while Parent/Child are classes...
see the answer below, may clarify your doubts.
class Parent():
def __init__(self):
self.myvar = 1
class Child(Parent):
def __init__(self):
Parent.__init__(self)
# here you can access myvar like below.
print self.myvar
child = Child()
print child.myvar
Solution 2
Parent does not have an attribute called myvar. Only instances of parent have that attribute. From within a method of Child, you can access that attribute with self.myvar
.
Solution 3
Alternative to using inheritance
The current answers are coming from an inheritance perspective, but this isn't always what you want -- sometimes you might want the child to be an entirely different type of object to the parent, but that still has access to the parent attributes.
For a business analogue, think of Excel Workbooks which have Worksheet children, which themselves have Range children, and so on.
Only Child
An alternative approach (and not the only one) is to pass the parent as an argument to the child to create a property that corresponds to the parent:
class Parent(object):
def __init__(self, parent_value):
self.parent_value = parent_value
self.child = Child(self)
class Child(object):
def __init__(self, _parent):
self.parent = _parent
self.child_value = 0
new_parent = Parent(1)
print(new_parent.parent_value) # prints 1
new_child = new_parent.child
print(new_child.child_value) # prints 0
print(new_child.parent.parent_value) # prints 1
new_parent.parent_value = 100
print(new_child.parent.parent_value) # prints 100
Note that this instantiates the child
at the same that that new_parent
is instantiated. To access the parent's attributes, just go through the parent
property.
Multiple Children
You could extend this so that you can create multiple instances of the Child
class through the new_parent
object. The code below is one simple way of doing this which replaces the child
property with a children
property and an add_child
method.
class Parent(object):
def __init__(self, parent_value):
self.parent_value = parent_value
self.children = []
def add_child(self, child_value):
new_child = Child(child_value, _parent=self)
self.children.append(new_child)
return new_child # allows add_child to assign a variable
class Child(object):
def __init__(self, child_value, _parent):
self.parent = _parent
self.child_value = child_value
new_parent = Parent(1)
# add 3 Child instances with child_values 2, 4, 8
[new_parent.add_child(v) for v in [2, 4, 8]]
# add another child that utilises the return statement
extra_child = new_parent.add_child(16)
for child in new_parent.children:
print(child.child_value) # prints 2, 4, 8, 16
print(child.parent.parent_value) # prints 1
new_parent.parent_value = 32
for child in new_parent.children:
print(child.parent.parent_value) # prints 32
# prove that extra_child is new_parent.children[3]
extra_child.child_value = 64
print(new_parent.children[3].child_value) # prints 64
Related videos on Youtube
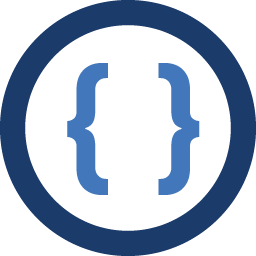
Admin
Updated on August 10, 2022Comments
-
Admin over 1 year
How to access "myvar" from "child" in this code example:
class Parent(): def __init__(self): self.myvar = 1 class Child(Parent): def __init__(self): Parent.__init__(self) # this won't work Parent.myvar child = Child()
-
ihadanny about 3 yearsif your attribute starts with a 'dunder' (e.g.
self.__myvar
) it won't work as python considers it private
-
-
Admin almost 12 yearsI could have sworn I tried the normal "self" instance reference. Oh well. Waiting to accept answer...
-
Ahmed Alhallag over 3 yearsNote: in this case, you don't need to have an init method in the subclass for this solution to work.
-
Bill Wallis about 3 yearsFor future readers, the
super()
method is preferred in the child class__init__
instead of the parent class name. See this question -
Peilonrayz about 3 yearsWhilst your answer is good quality, I don't think your answer answers the question as is. The question asks about inheritance not about composition or container classes. And I honestly don't think the OP needs to employ composition here.