How to get Python Object Parent?
Solution 1
(I know this is an old question but since it's unanswered...)
You could try messing with the garbage collector. Was going to suggest looking at the module level objects but some quick code experimentation gave this: Try the gc.get_referrers(*objs)
function.
import gc
class Foo(object):
"""contains Bar"""
def __init__(self, val):
self.val = val
self.bar = None
class Bar(object):
"""wants to find its parents"""
def __init__(self, something):
self.spam = something
def find_parents(self):
return gc.get_referrers(self)
The results aren't straightforward so you'll have to apply your own logic for how determine which f
is the one you're looking for. Notice the 'f': <__main__.Foo object at ...>
below:
>>> f = Foo(4)
>>> b = Bar('ham')
>>> f.bar = b
>>> b.find_parents()
[{'bar': <__main__.Bar object at 0x7f3ec5540f90>, 'val': 4}, <frame object at 0x238c200>,
{'b': <__main__.Bar object at 0x7f3ec5540f90>,
'Bar': <class '__main__.Bar'>,
'f': <__main__.Foo object at 0x7f3ec5540f10>, # <-- these might be
# the droids you're looking for
'__builtins__': <module '__builtin__' (built-in)>,
'__package__': None, 'gc': <module 'gc' (built-in)>, '__name__': '__main__',
'Foo': <class '__main__.Foo'>, '__doc__': None
}]
For bonus points, use gc.get_referents(*objs)
on those to check if the same b
is in their list.
Some notes/caveats:
- I think you should just pass the parent Foo object to Bar, either in
init
or another method. That's what I would do, instead of my suggestion above. - This SO answer points out some issues the garbage collector has when linking both ways - you're not currently doing that but if you do, fyi.
Solution 2
you could do something like this:
class Object:
def __setattr__(self, name, value):
if isinstance(value, Test):
value.set_parent(self)
self.__dict__[name] = value
class Test:
def set_parent(self, parent):
self.parent = parent
o = Object()
o.test = Test()
assert o.test.parent is o
Solution 3
well, I donno what exactly you are trying. but I have done something like this.
class Node(object):
def __init__(self, name):
self.parent = ''
self.child = []
self.name = name
def setParent(self, parent):
if isinstance(parent, tempNode):
self.parent = parent.name
parent.setChild(self.name)
def setChild(self, child):
self.child.append(child)
def getParent(self):
return self.parent
def getChild(self):
return self.child
and to make a parent you can say
n1 = Node('Father')
n2 = Node('Son')
n2.setParent('Father') # it will set n1 as parent and n2 as child at the same time.
Hope this will help you.
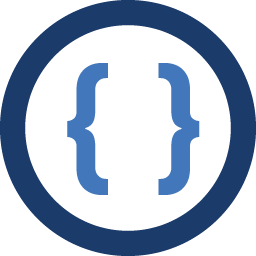
Admin
Updated on December 20, 2021Comments
-
Admin over 2 years
So, I'm trying to get the object that a custom object is 'inside' of. Here's an example.
Assume that o is an object - it doesn't matter what kind, it can just store variables.
o = Object() class Test(): def __init__(self): self.parent = o ## This is where I fall off; I want to be able to access ## the o object from within the Test object o.test = Test()
So, how would I get o from inside of Test? I know I could write the Test function to pass it in with
o.test = Test(o)
but I'd rather do it from inside the class definition.
-
phant0m almost 13 yearsHe didn't refer to his code as inheritance. He just showed that he (the OP) could just access
o
viaself.parent
from within the object, because that's what he asked for, most likely. -
krasnerocalypse almost 13 yearsWhat phant0m said. The way he's describing his need as "the object that a custom object is 'inside' of," I don't think inheritance is what he wants.
-
Admin almost 13 yearsThey're not inheritable at all - the two classes have nothing to do with each other. The point is just to get the object that an object of a class is stored in - basically, given objectA.obj = ObjectB(), is there a way to get objectA from within ObjectB's code, like a owner property or something like that? Or do I have to do it myself?
-
Admin almost 13 yearsNo, like kasnero and phant0m said, my question doesn't have to do anything with inheritance, but rather an issue of getting an object from within another object.
-
Dan D. almost 13 yearsthe problem with that is there is no way for
Test()
to react to being assiged to a nametest
on another objecto
other than for that objecto
to react to the assignment too.test
and for it to tell the assigned objectTest()
. -
velis over 9 years@SolarLune: you can reference an object a gazillion times, e.g.
a = b = c = obj
. Which of those three would .owner return? You DO havegc.get_referrers(*objs)
though which (maybe) does what you want. Edit: oops, this is OLD. Disregard.