Add alpha support to fragment shader (GLSL)
Well, your change to the fragment program discards the whole fragment if the color in _tex1
is transparent. That won't have the desired effect. What you should do is to back out your change and simply use float4
instead of float3
for the color variables and remove the .xyz
at the end of the assignment. That is:
float4 oricol = tex2D (_tex0,float2(q.x,q.y));
float4 col = tex2D (_tex1,float2(i.uv.x,i.uv.y));
But the whole program can be simplified a lot. Something like the following should be equivalent (untested):
half4 frag (v2f i) : COLOR {
float4 oricol = tex2D(_tex0, i.uv);
float4 col = tex2D(_tex1, i.uv);
float animtime = _Time * 10.0;
float comp = smoothstep(0.2, 0.7, sin(animtime));
float coeff = clamp(-2.0 + 2.0 * i.uv.x + 3.0 * comp, 0.0, 1.0);
float4 result = lerp(col, oricol, coeff);
return result;
}
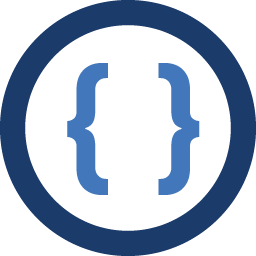
Admin
Updated on July 26, 2022Comments
-
Admin almost 2 years
I got the code from here. This shader cycles through 2 textures over time, with a "wipe" effect (see source for a demo). Now that I've learned and kind of understand most of it, I'm trying to change it to be able to use textures with alpha.
Here's the shader code :
Shader "Wipe" { Properties{ _tex0 ("Texture1", 2D) = "white" {} _tex1 ("Texture2", 2D) = "white" {} } SubShader{ Tags {"Queue"="Geometry"} Pass{ CGPROGRAM #pragma target 3.0 #pragma vertex vert #pragma fragment frag #include "UnityCG.cginc" sampler2D _tex0; sampler2D _tex1; float4 _tex0_ST; struct v2f { float4 pos : POSITION; float4 color : COLOR0; float4 fragPos : COLOR1; float2 uv : TEXCOORD0; }; v2f vert (appdata_base v){ v2f o; o.pos = mul (UNITY_MATRIX_MVP, v.vertex); o.fragPos = o.pos; o.uv = TRANSFORM_TEX (v.texcoord, _tex0); o.color = float4 (1.0, 1.0, 1.0, 1); return o; } half4 frag (v2f i) : COLOR{ float animtime = _Time*10.0; float2 q = i.uv.xy / float2(1,1); float3 oricol = tex2D (_tex0,float2(q.x,q.y)).xyz; float3 col = tex2D (_tex1,float2(i.uv.x,i.uv.y)).xyz; float comp = smoothstep( 0.2, 0.7, sin(animtime) ); col = lerp(col,oricol, clamp(-2.0+2.0*q.x+3.0*comp,0.0,1.0)); // custom alpha bit float4 textureColor = float4(col,1); if(textureColor.a < 1.0){ discard; } return textureColor; } ENDCG } } //FallBack "VertexLit" }
I tried to implement a small code snippet in the last function for alpha support. I tried changing Tags to {"Queue"="Transparent" "IgnoreProjector"="True" "RenderType"="Transparent"}, setting Cull and ZWrite to Off, adding Blend SrcAlpha OneMinusSrcAlpha... basically tried discarding transparent fragments, alpha testing, and blending, but I must be doing something wrong.
I set my two textures (which are PSDs, Photoshop file format with Alpha) to "Advanced" Texture Type, with "Generate Mip Maps" turned off, "Alpha is Transparency" turned on, and "Format" to "RGBA 32 bit". With these settings and the shader above, my textures preview as they should, but my textured plane is black...
Anyone could either help me out or tip me in the right direction?
EDIT : (sorry for the links, I don't have enough reputation yet to post them all)
This is what I get with the unedited shader from here + PSD set to normal (no advanced texture settings) : http: //answers.unity3d.com/storage/temp/22441-unity_base.jpg
This is what I get with current shader (code above) + a PSD as a texture, set to RGBA 32-bit, no Mip Maps, and 'Alpha is Transparency' turned on : http: //answers.unity3d.com/storage/temp/22442-unity_black.jpg
And this is what I'm trying to achieve (but not with a still image, I want to keep the original "Wipe" effect) : http: //nicolasraspail.com/unity_achieve.png
EDIT : Here's the shader working properly (thanks @nwellnhof !)
Shader "Wipe" { // Editor controllers Properties{ _tex0 ("Texture1", 2D) = "white" {} _tex1 ("Texture2", 2D) = "white" {} } SubShader{ Tags {"Queue"="Transparent" "IgnoreProjector"="True" "RenderType"="Transparent"} ZWrite Off Blend SrcAlpha OneMinusSrcAlpha Pass{ CGPROGRAM #pragma target 3.0 #pragma vertex vert #pragma fragment frag #include "UnityCG.cginc" sampler2D _tex0; sampler2D _tex1; float4 _tex0_ST; struct v2f { float4 pos : POSITION; float4 color : COLOR0; float4 fragPos : COLOR1; float2 uv : TEXCOORD0; }; v2f vert (appdata_base v){ v2f o; o.pos = mul (UNITY_MATRIX_MVP, v.vertex); o.fragPos = o.pos; o.uv = TRANSFORM_TEX (v.texcoord, _tex0); o.color = float4 (1.0, 1.0, 1.0, 1); return o; } half4 frag (v2f i) : COLOR{ float4 oricol = tex2D(_tex0, i.uv); float4 col = tex2D(_tex1, i.uv); float animtime = _Time * 10.0; float comp = smoothstep(0.2, 0.7, sin(animtime)); float coeff = clamp(-2.0 + 2.0 * i.uv.x + 3.0 * comp, 0.0, 1.0); float4 result = lerp(col, oricol, coeff); return result; } ENDCG } } //FallBack "VertexLit" }
-
Admin about 10 yearsThanks for your answer. Your frag function is actually working, but I get the exact same results as before (this). Could it be an image format or import issue ? I tried PSD (Photoshop CS6), PNG (doesn't import as expected), TGA (identical result as PSD). For each of them, transparency shows just like on my screenshot...
-
nwellnhof about 10 yearsIf the texture works correctly with one of the built-in transparent shaders (for example, Transparent Diffuse), then it's not an import issue. Also note that you still have to make the changes to Tags, ZWrite, and Blend that you mentioned.
-
Admin about 10 yearsThanks a lot ! I didn't realize I had to include previous tags, zwrite and blending options.