add NSTimeInterval to NSDate in cocoa
Solution 1
Have you seen the Dates and Times Programming Topics for Cocoa manual?
Basically you need to convert your time into an NSDate. To convert a string to a date you use the NSDateFormatter
class or maybe NSDateComponents
if you already know the hours, minutes and seconds.
Your NSTimeInterval
is just a double (i.e., 3600.0).
Then you use the dateByAddingTimeInterval
method of NSDate to add the number of seconds to the time.
NSDate* newDate = [oldDate dateByAddingTimeInterval:3600.0];
You can then use either NSDateFormatter
or NSDateComponents
to get the new time back out again.
Solution 2
addTimeInterval is now deprecated. Try using dateByAddingTimeInterval instead.
Solution 3
// This will set the time when your view appears it will also
// set the time if you want to retrieve from it.
-(void)viewDidAppear:(BOOL)animated
{
//Create todays date
NSDate *duedate = [NSDate date];
//Add how ever many days you could write 86400*numbe_of_days days 86400/24 for an hour
//Then simply reassign the variable or use a different one.
duedate = [duedate dateByAddingTimeInterval:86400]; //I add one day
[datePicker setDate:duedate animated:YES]; //Animate yes will show your pickers moving
[datePicker setMinimumDate:duedate]; //Set minimum date.
}
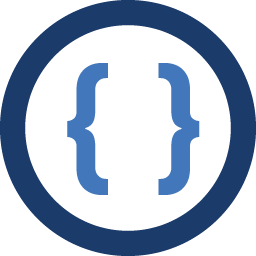
Admin
Updated on October 26, 2020Comments
-
Admin over 3 years
I've got a
NSDate
that represents a specific time. The format of that date is hhmmss. I want to add anNSInterval
value (specified in seconds) to that time value.Example:
NSDate = 123000 NSTimeInterval = 3600 Added together = 133000
What is the best way to do this?