Adding string objects to NSMutableArray?
Solution 1
tempString
isn't actually a string literal. @"Planet_%03d"
is a string literal. tempString
is an autoreleased object, meaning that it will be released when the NSAutoreleasePool is drained. Basically, the memory is already managed and you don't have to do anything.
The rule is: If you new
, alloc
, copy
or retain
an object, then you have to release
it. Otherwise, the memory is already managed, probably by an autorelease.
Also, you forgot to release pool
. Other than that, it looks fine.
Solution 2
One possible reason for the "unable to read unknown load command 0x80000022" error appears to be that I've upgraded to Snow Leopard without upgrading the developers tools at the same time. It looks like the error might be caused by trying to use the 10.5 version to XCode to compile in a 10.6 environment. I will look into this tomorrow.
Xcode 3.2 is now available in the Snow Leopard (Mac OS X 10.6) release. After installing Snow Leopard, upgrade to Xcode 3.2 by installing it separately from the Xcode Tools disk image. You can install it over prior versions of Xcode, or move them aside prior to installing.
PS: When I got the "unable to read unknown load command 0x80000022" error I was running OSX 10.6.1 with xCode 3.1.2
cheers -gary-
Related videos on Youtube
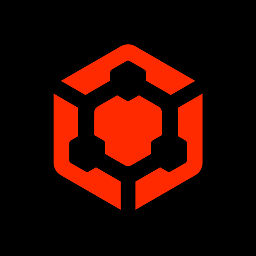
fuzzygoat
Apple Development @ Fuzzygoat, A digital nomad adventuring in one possible future.
Updated on June 04, 2022Comments
-
fuzzygoat 7 months
I have a little foundation tool test (Objective-C) that I am playing with and I have a few questions ...
#import <Foundation/Foundation.h> int main (int argc, const char * argv[]) { NSAutoreleasePool * pool = [[NSAutoreleasePool alloc] init]; int planetLoop; NSString *tempString; NSMutableArray *planetArray = [[NSMutableArray alloc] init]; NSLog(@"STRING ARRAY ... Start"); for(planetLoop=0; planetLoop<10; planetLoop++) { tempString = [NSString stringWithFormat: @"Planet_%03d", planetLoop+1]; NSLog(@"Planet_%03d", planetLoop+1); [planetArray addObject:tempString]; } [planetArray release]; [pool drain]; return 0; }
First, usually I release an object after adding it to an array, but am I right in thinking that what I have currently is right because "tempString" is a string literal, and as such does not need to be allocated or released?
Secondly, when I run this (prior to execution) I get the following eror "unable to read unknown load command 0x80000022" if this a problem with my code? from searching on google it looks like it might be a bug in xCode 3.1.2, anyone have any ideas?
Finally anything I am doing wrong, the idea is to fill an array with 10 string "Planet_001" through to "Planet_010"
EDIT: Ah, I see, thats because of the "= [NSString" bit i.e.
// Autoreleased object tempString = [NSString stringWithFormat: @"Planet_%03d", planetLoop+1]; // String literal tempString = @"Planet_";
many thanks, much appreciated -gary-
-
NSResponder over 13 yearsIf your tools aren't in sync with the OS version you're using, fix that first. It's a known unstable configuration.
-
Chris Suter over 13 yearsHe does release the pool: [pool drain]
-
Tom Dalling over 13 years@Chris you're right. I wasn't aware that
drain
does arelease
.