Android Battery in SDK
Solution 1
You can register an Intent receiver to receive the broadcast for ACTION_BATTERY_CHANGED: http://developer.android.com/reference/android/content/Intent.html#ACTION_BATTERY_CHANGED. The docs say that the broadcast is sticky, so you'll be able to grab it even after the moment the battery state change occurs.
Solution 2
Here is a quick example that will get you the amount of battery used, the battery voltage, and its temperature.
Paste the following code into an activity:
@Override
public void onCreate() {
BroadcastReceiver batteryReceiver = new BroadcastReceiver() {
int scale = -1;
int level = -1;
int voltage = -1;
int temp = -1;
@Override
public void onReceive(Context context, Intent intent) {
level = intent.getIntExtra(BatteryManager.EXTRA_LEVEL, -1);
scale = intent.getIntExtra(BatteryManager.EXTRA_SCALE, -1);
temp = intent.getIntExtra(BatteryManager.EXTRA_TEMPERATURE, -1);
voltage = intent.getIntExtra(BatteryManager.EXTRA_VOLTAGE, -1);
Log.e("BatteryManager", "level is "+level+"/"+scale+", temp is "+temp+", voltage is "+voltage);
}
};
IntentFilter filter = new IntentFilter(Intent.ACTION_BATTERY_CHANGED);
registerReceiver(batteryReceiver, filter);
}
On my phone, this has the following output every 10 seconds:
ERROR/BatteryManager(795): level is 40/100 temp is 320, voltage is 3848
So this means that the battery is 40% full, has a temperature of 32.0 degrees celsius, and has voltage of 3.848 Volts.
Solution 3
public static String batteryLevel(Context context)
{
Intent intent = context.registerReceiver(null, new IntentFilter(Intent.ACTION_BATTERY_CHANGED));
int level = intent.getIntExtra(BatteryManager.EXTRA_LEVEL, 0);
int scale = intent.getIntExtra(BatteryManager.EXTRA_SCALE, 100);
int percent = (level*100)/scale;
return String.valueOf(percent) + "%";
}
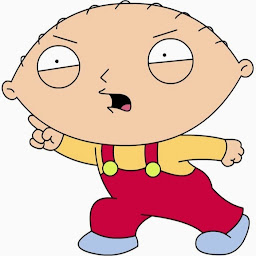
Roo
I've heard of Compute Engine, .NET, and Android. I work at Google as a Software Engineer. I currently work on Google Compute Engine. The answers I post are of my own opinion, they may be incorrect or outdated and do not reflect those of my employer.
Updated on August 17, 2022Comments
-
Roo over 1 year
Is there a way to get battery information from the Android SDK? Such as battery life remaining and so on? I cannot find it through the docs.