Android - Difference between Gridlayout and Staggered Gridlayout
Solution 1
Grid View : It is is a ViewGroup
that displays items in a two-dimensional, scrollable grid. In this each Grid is of same size (Height and width). Grid View shows symmetric items in view.
Staggered Grid View : It is basically an extension to Grid View
but in this each Grid is of varying size(Height and width). Staggered Grid View shows asymmetric items in view.
Tutorial to implement Staggered Grid View :
Solution 2
My time at Oodles Technologies taught me about staggered. I'll share that.
StaggeredGridLayout is a LayoutManager, it is just like a GridView but in this grid each view have its own size (height and width). It supports both vertical and horizontal layouts.
Below are some basic steps to create a staggered grid:
1) Create a view.
As we know StaggeredGrid is not a direct view, it is a LayoutManager that lays out children in a staggered grid formation. We use RecyclerView as a view for the staggerd grid. Here is our RecyclerView in layout:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<android.support.v7.widget.RecyclerView
android:id="@+id/favPlaces"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</RelativeLayout>
2) Set StaggeredGridLayout LayoutManager.
Once our view is ready, let's use LayoutManager to create grids on the view:
RecyclerView favPlaces = (RecyclerView) findViewById(R.id.favPlaces);
StaggeredGridLayoutManager layoutManager = new StaggeredGridLayoutManager(2,StaggeredGridLayoutManager.VERTICAL);
layoutManager.setGapStrategy(StaggeredGridLayoutManager.GAP_HANDLING_NONE);
favPlaces.setLayoutManager(layoutManager);
favPlaces.setHasFixedSize(true);
3) Adapter to inflate the StaggeredGrid views.
To inflate the data in form of a grid, we first need a layout which will represent that data. We are using CardView for this and the layout is:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<android.support.v7.widget.CardView
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:cardcornerradius="4dp"
app:cardusecompatpadding="true">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/colorPrimary"
android:orientation="vertical">
<ImageView
android:id="@+id/placePic"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:adjustviewbounds="true"
android:scaletype="fitXY" />
<TextView
android:id="@+id/placeName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:textsize="16sp" />
</LinearLayout>
</android.support.v7.widget.CardView>
</LinearLayout>
After we setup our all the basic steps, it's time to complete our main activity. Here is the complete code of MainActivity:
public class MainActivity extends AppCompatActivity {
int placeImage[]= {R.drawable.agattia_airport_lakshadweep,R.drawable.nainital,R.drawable.goa,
R.drawable.lotus_temple,R.drawable.valley_of_flowers,R.drawable.ranikhet,R.drawable.dehradun,R.drawable.nainital1};
String placeName[]= {"Lakshadweep, India","Nainital, India","Goa, India","Lotus-Temple, India","Valley-Of-Flowers, India","Ranikhet, India",
"Dehradun, India","Nainital, India"};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
RecyclerView favPlaces = (RecyclerView) findViewById(R.id.favPlaces);
StaggeredGridLayoutManager layoutManager = new StaggeredGridLayoutManager(2,StaggeredGridLayoutManager.VERTICAL);
layoutManager.setGapStrategy(StaggeredGridLayoutManager.GAP_HANDLING_NONE);
favPlaces.setLayoutManager(layoutManager);
favPlaces.setHasFixedSize(true);
ArrayList<PlaceDetails> placeList = getPlaces();
StaggeredAdapter staggeredAdapter = new StaggeredAdapter(placeList);
favPlaces.setAdapter(staggeredAdapter);
}
private ArrayList<PlaceDetails> getPlaces() {
ArrayList<PlaceDetails> details = new ArrayList<>();
for (int index=0; index<placeImage.length;index++){
details.add(new PlaceDetails(placeImage[index],placeName[index]));
}
return details;
}
}
Solution 3
StaggeredGridlayout
- This lays out children in a staggered grid formation.
- It supports horizontal & vertical layout as well as an ability to layout children in reverse.
- Staggered grids are likely to have gaps at the edges of the layout.
- To avoid the gaps,
StaggeredGridLayoutManager
can offset spans independently or move items between spans. You can control this behavior viasetGapStrategy(int)
.
GridLayout
- This lays out its children in a rectangular grid.
- The grid is composed of a set of infinitely thin lines that separate the viewing area into cells.
- Children occupy one or more contiguous cells, as defined by their
rowSpec
andcolumnSpec
layout parameters.
Solution 4
Grid Layout (API Level 14): A layout that places its children in a rectangular grid.
The number of rows and columns within the grid can be declared using the android:rowCount
and android:columnCount
properties. Typically, however, if the number of columns is declared the GridLayout will infer the number of rows based on the number of occupied cells making the use of the rowCount property unnecessary.
Similarly, the orientation of the GridLayout may optionally be defined via the android:orientation
property.
I think there is no separate StaggeredGridLayout. Here are the things we do have
StaggeredGridLayoutManager : It is one of the a layout manager used in Recyclerview.A LayoutManager that lays out children in a staggered grid formation. It supports horizontal & vertical layout as well as an ability to layout children in reverse.
Staggered GridView : The StaggeredGridView allows the user to create a GridView with uneven rows similar to how Pinterest looks. Includes own OnItemClickListener and OnItemLongClickListener, selector, and fixed position restore.Please look at this example.
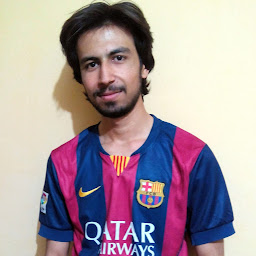
IBRAR AHMAD
Updated on July 09, 2022Comments
-
IBRAR AHMAD almost 2 years
I am working in android material design api & want to display some data in grid format. I tried both
GridLayout
andStaggeredGridlayout
and both look same. For general information, i want to ask what is the difference betweenGridlayout
andStaggeredGridlayout
?
Thank you. -
java acm over 7 yearsyour image is not StaggeredGridLayout , it's custom GridLayout from github.com/felipecsl/AsymmetricGridView
-
Skaldebane about 4 years@javaacm it doesn't really matter as he's clarifying his point correctly...