Android ListView in Fragment
Solution 1
It seems like your Fragment should subclass ListFragment (not ListActivity). onCreateView()
from ListFragment will return a ListView you can then populate.
Here's more information on populating lists from the developer guide: Hello, ListView
Solution 2
Here's a code snippet to help you display ListView in a fragment.:
public class MessagesFragment extends ListFragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_messages, container,
false);
String[] values = new String[] { "Message1", "Message2", "Message3" };
ArrayAdapter<String> adapter = new ArrayAdapter<String>(getActivity(),
android.R.layout.simple_list_item_1, values);
setListAdapter(adapter);
return rootView;
}
}
And, this is how the xml would look like:
<ListView
android:id="@android:id/list"
android:layout_width="match_parent"
android:layout_height="wrap_content" >
</ListView>
If you do not use 'list' as ID or do not include a ListView into your layout, the application crashes once you try to display the activity or the fragment.
Reference: http://www.vogella.com/tutorials/AndroidListView/article.html#listfragments
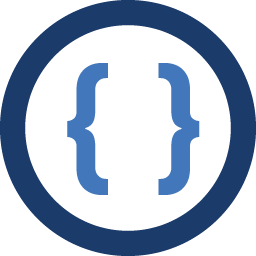
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am trying to create a list of items which contains an image and some description for the image in each individual. After which, the list will be place in a
fragment
inside the application. Can anyone guide me to create it? I am not too sure how can I do it without theListActivity
. -
citizen conn almost 13 yearsIf you found this answer helpful, then mark as the answer and post a new question for your new problem that someone can help you with.
-
Victor R. Oliveira over 7 yearsmake sure to not wrap_content in ListView for layout_height, instead fill_parent or match_parent.stackoverflow.com/questions/11295080/…