Android Room error: Dao class must be annotated with @Dao
Solution 1
Check your database class. When you define DAO, you must have use wrong type(Device instead of DeviceDAO).
Incorrect
public abstract Device deviceDao();
Correct
public abstract DeviceDAO deviceDao();
Hope this will work. Thanks
Solution 2
Error Message: Dao class must be annotated with @Dao
To solve error please read it properly.
If this error messages shows on Model class then you need to modify your AppDatabase class. I am giving you the code what gives error then error corrected code.
Error Code:
MyImage.java
@Entity
public class MyImage {
@PrimaryKey(autoGenerate = true)
private int uid;
@ColumnInfo(name = "title")
private String title;
@ColumnInfo(name = "photo")
private String photo;
public MyImage(String title, String photo) {
this.title = title;
this.photo = photo;
}
public int getUid() {
return uid;
}
public void setUid(int uid) {
this.uid = uid;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getPhoto() {
return photo;
}
public void setPhoto(String photo) {
this.photo = photo;
}
}
MyImageDao.java
@Dao
public interface MyImageDao {
@Query("SELECT * FROM myimage")
List<MyImage> getAll();
@Insert
void insertAll(MyImage... myImages);
@Delete
void delete(MyImage myImage);
}
AppDatabase.java
@Database(entities = {MyImage.class}, version = 1)
public abstract class AppDatabase extends RoomDatabase {
public abstract MyImage myImageDao();
}
Here has error on only AppDatabase.java file, you can see myImageDao has return type MyImage, that means it assumed that MyImage is a Dao class but MyImage is model class and MyImageDao is Dao class. So it need to modify AppDatabase.java class and MyImage to MyImageDao.
The corrected code is-
AppDatabase.java
@Database(entities = {MyImage.class}, version = 1)
public abstract class AppDatabase extends RoomDatabase {
public abstract MyImageDao myImageDao();
}
Solution 3
For Kotlin users :
Check if you've added following line in your Database file.
abstract val myDatabaseDao:MyDatabaseDao
Solution 4
Check if you have any additional methods in your interface. In my Kotlin implementation I had:
@Dao interface DeviceDao {
@get:Query("SELECT * FROM extdevice")
val all: List<ExtDevice>
fun first() : ExtDevice? {
val devices = all
if (devices.isNotEmpty())
return devices[0]
return null
}
}
removing first() solved my issue:
@Dao interface DeviceDao {
@get:Query("SELECT * FROM extdevice")
val all: List<ExtDevice>
}
Solution 5
add
import androidx.room.Dao;
to your interface that u set querys on it and then add the first line from this code
@Dao
public interface UserDeo {
@Query("SELECT * FROM user")
List<User> getAllUsers();
@Insert
void insertAll(User... users);
}
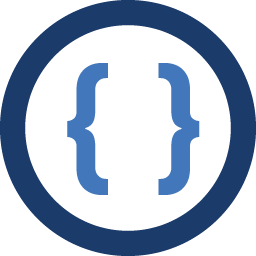
Admin
Updated on June 13, 2022Comments
-
Admin about 2 years
I'm using Room for my android app. I'm now trying to setup my database, but there is an error message, which says, that the Dao class must be annotated with @Dao. But as you can see in the coding snippet, the Dao class is annotated with @Dao. Does anyone know where the problem or my mistake could be? Both files aren't in the same folder (DAO is in the service folder while the other class is in the model folder)
Device.java
@Entity(tableName = "device") public class Device { @PrimaryKey(autoGenerate = true) public int device_id; @ColumnInfo(name = "identifier") public String identifier; @ColumnInfo(name = "language") public int language; @ColumnInfo(name = "searchFilter") public int searchFilter; public Device(String identifier, int language, int searchFilter){ this.identifier = identifier; this.language = language; this.searchFilter = searchFilter; } }
DeviceDAO.java
@Dao public interface DeviceDAO { @Insert(onConflict = OnConflictStrategy.REPLACE) void addDevicePreferences(DifficultType difficultType); @Query("SELECT * FROM device") List<Device> selectAllDevicePreferences(); @Update(onConflict = OnConflictStrategy.REPLACE) void updateDevicePreferences(Device device); }
-
Admin over 6 yearsI'm sure that I've wrote public abstract DeviceDao deviceDao(); Otherwise it would gave me an other error message. But I'm using now ObjectBox and it works. Anyhow thank you for your help.
-
Admin over 6 yearsThey were all imported and also have reimport them. And after this didn't work I have tried to rebuild my project but this didn't work, too. But now I'm using ObjectBox instead of Room and it works. Nevertheless thank you for your help.