Angular 6 calling function after dialog close
12,486
The error message pretty much says exactly what's wrong: the object you're returning from the method is not the correct type.
To fix this, we need to create and store off the new dialog, then create the subscription, and finally return the dialog object that we just created.
abrirModal(component: ComponentType<any>, dados: any, event: any = null): MatDialogRef<any, any> {
this.windowScrolling.disable();
// Create and store dialog object
const dialog = this.dialog.open(component, {minWidth: '90%', disableClose: true, data: dados, position: {top: '4%'}});
// Create subscription
dialog.afterClosed().subscribe(() => {
// Do stuff after the dialog has closed
this.windowScrolling.disable();
});
// Return dialog object
return dialog;
}
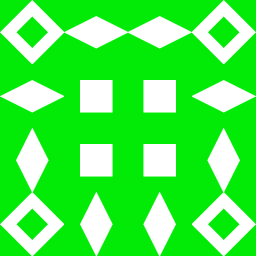
Author by
Estudante
Updated on December 06, 2022Comments
-
Estudante 11 months
I am trying to implement a function call after closing the dialog, but I can not use the pipe nor subscribe it. Let's see my code.
i got this error :
Type 'Observable<any>' is not assignable to type 'MatDialogRef<any, any>'. Property '_overlayRef' is missing in type 'Observable<any>'
the idea is to call the function
this.windowScrolling.disable ();
when to close the dialog@Injectable({ providedIn: 'root' }) export class NavegarService { public offsets: number[]; windowScrolling: WindowScrollingService; constructor(private router: Router, private activatedRoute: ActivatedRoute, windowScrolling: WindowScrollingService, public dialogRef: MatDialogRef <any, any>, private dialog: MatDialog) { this.windowScrolling = windowScrolling; this.offsets = this.range( 1, 20 ); } navegarContext(url: string, event: any = null) { this.router.navigate([url], {queryParams: {context: this.activatedRoute.snapshot.queryParams['context']}}); } navegar(url: string, event: any = null) { this.router.navigate([url]); } abrirModal(component: ComponentType<any>, dados: any, event: any = null): MatDialogRef<any, any> { this.windowScrolling.disable(); return this.dialog.open(component, {minWidth: '90%', disableClose: true, data: dados, position: {top: '4%'}}).afterClosed() .pipe(); // got error here } private range( from: number, to: number ): number[] { const values = []; for ( let i = from ; i <= to ; i++ ) { values.push( i ); } return( values ); } }