Angular - How to disable/grey out screen on load and save
Solution 1
What I ended up doing was simply adding a new CSS ID tag to the root CSS file (styles.css). This way I'm able to reference the ID tag from anywhere in my application in order to apply this to anything within my project.
I drive the toggling of the CSS element with a variable, this way I'm able to execute logic in order to toggle the grey/disable. The grey/disablement starts out on a button click, and ends when the save has completed from the database. The user is unable to edit any field on screen and is forced to wait for the completion of the save before modifying any more fields.
Here's the documentation that helped me achieve this: https://www.w3schools.com/howto/tryit.asp?filename=tryhow_css_overlay
Here's what I added to styles.css at the root project level:
#overlay {
position: fixed;
display: none;
width: 100%;
height: 100%;
top: 0;
left: 0;
right: 0;
bottom: 0;
background-color: rgba(0,0,0,0.5);
z-index: 2;
cursor: pointer;
}
Here's my HTML of my component where I applied the styling (I added this right at the bottom of my HTML file):
<div id="overlay">
<span id="nav" *ngIf="saveInProgress">
<div class="spinner" style="display: block; position: fixed">
</div>
</span>
<span id="nav" *ngIf="saveInProgress">
<div class="submit-message" style="display: block; color: #FFFFFF; position: fixed; left: 49.7%; top: 54.5%;">
Saving...
</div>
</span>
</div>
Here's my logic in the corresponding TS component:
save(): Observable<any> {
if(...) {
this.saveInProgress = true;
document.getElementById("overlay").style.display = "block";
}
....some more logic....
if(...){
this.saveInProgress = false;
document.getElementById("overlay").style.display = "none";
}
}
Solution 2
You can add the following css property to #nav (use div instead of span). This should work, as it will create an overlay over your website content so that the content is not accessible:
#nav {
background: #e9e9e9;
position: absolute;
top: 0;
right: 0;
bottom: 0;
left: 0;
opacity: 0.5;
}
Also, It would be very helpful if you provide a working example on a website like jsfiddle, stackblitz etc so that we can have a look at what it is you're trying to accomplish.
Update: Also, give this div an 'id' other than 'nav' so that the css does not conflict between your content and overlay.
Related videos on Youtube
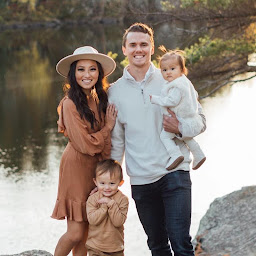
thenolin
Angular Enthusiast - Full Stack Angular/.NET Core Developer
Updated on June 04, 2022Comments
-
thenolin almost 2 years
I haven't found much out there specifically relating to Angular, but is there a way to grey out/disable the screen on a button click? Right now I have a button which is tied to saving some data, but I need to show a loading icon and not allow the user to edit any other fields while the save is in progress.
I currently have it set to where I can show the loading icon fine on the screen, I'm just wondering what I need to do so that the user isn't able to edit the DOM.
Right now I just have a spinner that is tied to an *ngIf, which displays towards the top of my page.
HTML:
<div id="nav"> <button type="submit" class="btn btn-primary" style="height: 46px; width: 188px; margin: 0 auto; display:block;" (click)="saveHandler()"> Save & Calculate </button> </div> <span id="nav" *ngIf="saveInProgress"> <div class="submit-spinner" style="margin-top: 20px; display:block"> </div> </span>
-
Marcel Hoekstra over 5 yearscan't you do something like this: stackoverflow.com/a/49534016/6729295
-
maxime1992 over 5 yearsI've made a lib that might helps you out. Check it out here github.com/maxime1992/ngx-hover-opacity (there's a stackblitz demo in the readme)
-
thenolin over 5 yearsI checked it out.. looked like it was pretty much what I needed.. imported it into my project but it ended up not working.. :(
-