AngularJs: pass $scope variable with service
Solution 1
You cannot inject $scope
dependency in service factory function.
Basically shareable service should maintain shareable data in some object.
Service
.service('share', function () {
var self = this;
//private shared variable
var sharedVariables = { };
self.getSharedVariables = getSharedVariables;
self.setVariable = setVariable;
//function declarations
function getSharedVariables() {
return sharedVariables;
}
function setVariable() {
sharedVariables[paramName] = value;
}
});
Controller
app.controller('Ctrl1', function ($scope, share) {
$scope.variable1 = "One";
share.setVariable('variable1', $scope.variable1); //setting value in service variable
});
app.controller('Ctrl2', function ($scope, share) {
console.log("shared variable " + share.getSharedVariables());
});
Solution 2
Yes, it is possible to share the scope variables between controllers through services and factory.But, the $scope variables are local to the controller itself and there is no way for the service to know about that particular variable.I prefer using factory, easy and smooth as butter. If you are using the service of factory in a separate file you need to include the file in index.html
app.controller('Ctrl1', function ($scope,myService,$state) {
$scope.variable1 = "One";
myService.set($scope.variable1);
$state.go('app.thepagewhereyouwanttoshare');//go to the page where you want to share that variable.
});
app.controller('Ctrl2', function ($scope, myService) {
console.log("shared variable " + myService.get());
});
.factory('myService', function() {
function set(data) {
products = data;
}
function get() {
return products;
}
return {
set: set,
get: get
}
})
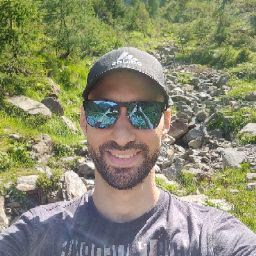
Giordano
Do you need help? contact me on Fiverr Follow me on: Twitter GitHub LinkedIn Programming: Python and Django HTML, CSS, UIkit and Bootstrap Javascript, AngularJS and JQuery Titanium Appcelerator OS: Linux (Debian, Ubuntu, Mint) Windows I'm interested in Raspberry Pi and embedded system.
Updated on June 28, 2022Comments
-
Giordano almost 2 years
I have two controllers and in one of them I declared a $scope variable that I would like visible in the second controller.
First controller
app.controller('Ctrl1', function ($scope) { $scope.variable1 = "One"; });
Second Controller
app.controller('Ctrl2', function ($scope, share) { console.log("shared variable " + share.getVariable()); });
I researched the best Angular approach and I found that is the use of service. So I added a service for
Ctrl1
Service
.service('share', function ($scope) { return { getVariable: function () { return $scope.variable1; } }; });
This code return this error:
Unknown provider: $scopeProvider <- $scope <- share
So my question is: is possible share $scope variable between controllers? Is not the best Angular solution or i'm missing something?
Sorry for my trivial question but I'm an Angular beginner.
Thanks in advance
Regards
-
Beyers about 8 yearsPolluting $rootScope is considered bad practice, similar to why global variables is considered bad practice. Use service/factory instead.
-
Jonas about 8 yearsYes that's true. But i think that this is what he asked for. In some cases it's usefull though
-
Beyers about 8 yearsI think the OP question was specifically around how to share data between controllers using a
service
.