Anonymous functions in WordPress hooks
Solution 1
The disadvantage of the anonymous function is that you're not able to remove the action with remove_action.
Important: To remove a hook, the $function_to_remove
and $priority
arguments must match when the hook was added. This goes for both filters and actions. No warning will be given on removal failure.
Because you didn't define function_to_remove
, you can't remove it.
So you should never use this inside plugins or themes that somebody else might want to overwrite.
Solution 2
Using closures has the benefit of keeping the global namespace clean, because you don't have to create a global function first to pass as a callback.
add_action('admin_init', function () {
// some code...
});
Personally I would prefer using closures as callbacks, unless:
- You want the possibility of removing the callback
- The callback function needs to be used more then once
- You need support for older PHP versions (less then 5.3)
Closures in Classes
Closures can also be beneficial within classes.
class SomeClass
{
public function __construct()
{
add_action('wp_head', function () {
$this->addSomeStyling();
});
}
protected function addSomeStyling()
{
echo '<style> body { color: #999; } </style>';
}
}
Normally callback methods need to be made public, but in this case you can also make them private or protected.
This solution only works for PHP 5.4+. To also make it work for PHP 5.3, you need to explicitly pass the $this
object reference to the closure, like:
public function __construct()
{
$self = $this;
add_action('wp_head', function () use ($self) {
$self->addSomeStyling();
});
}
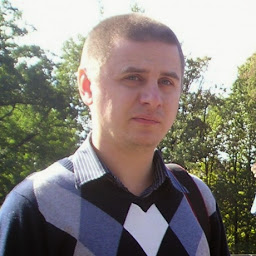
Aleksandr Levashov
Updated on June 04, 2022Comments
-
Aleksandr Levashov almost 2 years
WordPress hooks can be used in two ways:
using callback function name and appropriate function
add_action( 'action_name', 'callback_function_name' ); function callback_function_name() { // do something }
using anonymous function (closure)
add_action( 'action_name', function() { // do something } );
Is there any difference for WordPress what way to use? What is prefered way and why?
-
Admin over 7 yearsTotally disagree about using closures in hooks. You can never know how hooks may be handled by others. One, for example, may have the necessity to remove your hook and using closures he/she's stuck . Closures are a good thing only when used in a context where you're not manipulating a public API interface.
-
EFC about 7 yearsYou can remove even anonymous hooks using the
remove_all_actions()
andremove_all_filters()
. But even so, I agree that defining a regular function is the preferred pattern in WordPress. -
Peter Ajtai about 6 yearsYou can use namespaced functions the other way too:
add_action('acf/init', 'child_theme\load_content_types');
You would use this below anamespace child_theme;
. -
dingo_d over 4 yearsWord of advice, when you use anonymous functions for hooks callback, you are virtually making your code untestable. There's no way to even check the
has_action
for such callbacks, let alone making code covered. But code coverage is a different beast altogether... -
Arek Kostrzeba over 3 yearsInteresting article about subject: inpsyde.com/en/remove-wordpress-hooks
-
DevelJoe over 3 yearsJust came across this post and I really just wanted to emphasize against what has been stated in the comments to this answer. As stated in the answer of @TeeDeeJee, you should avoid this if your plugin / theme's supposed to be used / modified by others. But let's say you developed a main custom plugin on your own, which you only use for your own website, and the normal functioning of that plugin is vital to your website. In these cases, is it really a bad idea to make it more difficult to remove your (vital) plugin callbacks from hooks via anonymous functions? ....