Argument of type '{header:Header;}' is not assignable to parameter of type 'RequestOptionsArgs'
30,334
I finally solved mine by skipping the RequestOptions object.
I'm using Angular 4 so it may be different for me, but I create an untyped object and pass it into the http method function... -
import { Identifiable, RestRepositoryService, HalResponse } from '../spring-data/rest-repository.service';
import { Injectable } from '@angular/core';
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { ContentTemplate } from '../content-template/content-template.service';
export class ContentItem extends HalResponse {
id: string;
name: string;
description?: string;
fieldValues: any;
template: any;
contentType: any;
}
@Injectable()
export class ContentItemService extends RestRepositoryService<ContentItem> {
getContentType(contentItem: ContentItem) {
return this.http.get(contentItem._links.contentType.href);
}
getContentTemplate(contentItem: ContentItem) {
return this.http.get(contentItem._links.template.href);
}
setContentTemplate(contentItem: ContentItem, template: ContentTemplate) {
const headers = new HttpHeaders({'Content-Type': 'text/uri-list'});
return this.http.put(contentItem._links.template.href, template._links.self.href, {headers: headers});
}
constructor(http: HttpClient) {
super(http, '/api/contentItems');
}
}
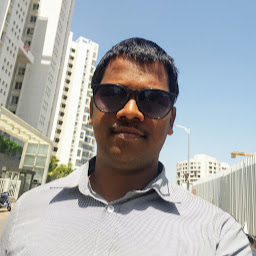
Author by
Gawade Pavan
Updated on December 02, 2020Comments
-
Gawade Pavan over 3 years
Im Getting following error while trying to pass header in HTTP.POST/GET request
Argument of type '{header:Header;}' is not assignable to parameter of type 'RequestOptionsArgs'. Property 'null' is missing in the type '{header:Header;}'
I have try many solutions but no lock on it.
here my code :
import { Injectable } from '@angular/core'; import { Response, RequestOptions, Headers, Http, URLSearchParams} from '@angular/http'; import { Observable, Subject } from 'rxjs/Rx'; import {Company} from './companyMaster'; import 'rxjs/add/operator/map'; import "rxjs/Rx"; import 'rxjs/add/operator/toPromise'; import {CommonService} from '../../common.service'; @Injectable() export class CompanyMasterService { private GetListActionUrl: string; private AddEditActionUrl: string; public headers = new Headers({ 'Content-Type': 'application/json' }); public options = new RequestOptions({ headers: this.headers }); commonService: CommonService; constructor(private _http: Http) { this.commonService = new CommonService(); this.GetListActionUrl = this.commonService.RootURL + 'Company/GetList'; this.AddEditActionUrl = this.commonService.RootURL + "Company/AddEdit"; } private RegenerateData = new Subject<number>(); // Observable string streams RegenerateData$ = this.RegenerateData.asObservable(); GetList(): Promise<Company[]> { return this._http.get(this.GetListActionUrl + "?branchId=" + this.commonService.BranchId) .toPromise() .then(response => this.extractArray(response)) .catch(); } AddEdit(company: Company): any { let headers = new Headers({ 'content-type': 'application/json' }); let body = JSON.stringify({ 'BRANCH_ID': company.BRANCH_ID.toString(), 'COMPANY_ID': company.COMPANY_ID != null ? company.COMPANY_ID.toString() : null, 'COMPANY_NAME': company.COMPANY_NAME }); let options = new RequestOptions({ headers: headers}); return this._http.post(this.AddEditActionUrl, body, options) .toPromise() .then(response => this.extractArray(response)) .catch(); } protected extractArray(res: Response, showprogress: boolean = true) { let data = res.json(); return data || []; } private getHeaders() { // I included these headers because otherwise FireFox // will request text/html instead of application/json let headers = new Headers(); headers.append('Accept', 'application/json'); return headers; }
}
See above code I have passed header in method AddEdit() but it shows above error i have try many solution like :
- Update Package
- Imported @angular/http
- Tried different way to pass header Tried this solution
i have attached screenshot showing error in Visual studio IDE. See attachement error screenshot please reply as soon as possible.
package.json
{ "name": "sb-admin-angular4-bootstrap4", "version": "1.0.0", "license": "MIT", "scripts": { "ng": "ng", "start": "ng serve --ec=true", "build": "ng build --prod", "gitbuild": "ng build --prod --base='/start-angular/SB-Admin-BS4-Angular-4/master/dist/'", "test": "ng test", "lint": "ng lint", "e2e": "ng e2e" }, "private": true, "dependencies": { "@angular/common": "^4.0.0", "@angular/compiler": "^4.0.0", "@angular/core": "^4.0.0", "@angular/forms": "^4.0.0", "@angular/http": "^4.0.0", "@angular/platform-browser": "^4.0.0", "@angular/platform-browser-dynamic": "^4.0.0", "@angular/router": "^4.0.0", "@ng-bootstrap/ng-bootstrap": "^1.0.0-alpha.25", "@ngx-translate/core": "^6.0.1", "@ngx-translate/http-loader": "0.0.3", "core-js": "^2.4.1", "font-awesome": "^4.7.0", "ionicons": "^3.0.0", "ng2-charts": "^1.5.0", "rxjs": "^5.1.0", "typing": "^0.1.9", "zone.js": "^0.8.4" }, "devDependencies": { "@angular/cli": "^1.1.3", "@angular/compiler-cli": "^4.0.0", "@types/jasmine": "2.5.38", "@types/node": "~6.0.60", "codelyzer": "~2.0.0", "jasmine-core": "~2.5.2", "jasmine-spec-reporter": "~3.2.0", "karma": "~1.4.1", "karma-chrome-launcher": "~2.0.0", "karma-cli": "~1.0.1", "karma-coverage-istanbul-reporter": "^0.2.0", "karma-jasmine": "~1.1.0", "karma-jasmine-html-reporter": "^0.2.2", "karma-phantomjs-launcher": "^1.0.4", "protractor": "~5.1.0", "ts-node": "~2.0.0", "tslint": "~4.5.0", "typescript": "~2.2.0", "webpack": "^3.0.0" } }
-
kelrob-dev about 4 yearsI had to look at your code line by line, what solved it for me was changing
headers = new Headers()
toheaders = new HttpHeaders()
-
Ms.KV about 4 years@kelrob- Yes it works by changing
new Headers()
tonew HttpHeaders()
.