how to add custom headers to httpRequest in angular
11,415
Solution 1
You should add header to your get request like this. Also since HttpHeaders is immutable object you have to reassign header object
public async AllCustomers(): Promise<ICourses[]> {
const apiUrl = `${this.baseUrl}/courses`;
let headers = new HttpHeaders();
headers = headers.append('Authorization', this.authKey);
headers = headers.append('x-Flatten', 'true');
headers = headers.append('Content-Type', 'application/json');
return this.http.get<ICourses[]>(apiUrl, {headers}).toPromise();
}
Solution 2
I think you forgot to add headers object in request
From
return this.http.get<ICourses[]>(apiUrl).toPromise();
To
return this.http.get<ICourses[]>(apiUrl, { headers }).toPromise();
Solution 3
import { HttpHeaders } from '@angular/common/http';
import { HttpClient } from '@angular/common/http';
constructor(private _http: HttpClient) {
}
public getHeaders(): HttpHeaders {
const headers = new HttpHeaders({
'Content-Type': 'application/json',
'Authorization': `Bearer ${this._auth.token}`
});
return headers;
}
public getData() {
const url = `https://stackoverflow.com/api/test`;
const body = { };
const options = { headers: this.getHeaders() };
return this._http.post<any>(url, body, options);
}
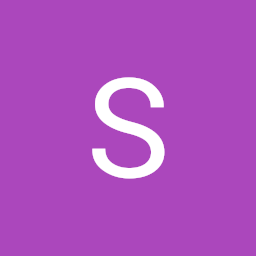
Author by
SGR
Updated on June 30, 2022Comments
-
SGR almost 2 years
I am trying make an
http get()
request by passing somevalues
inheaders
, Now i am replacing theheaders
like this:import { HttpClient, HttpHeaders } from '@angular/common/http'; import { Injectable } from '@angular/core'; import {ICustomer} from 'src/app/models/app-models'; @Injectable({ providedIn: 'root' }) export class MyService { private baseUrl = '....api url....'; public authKey = '....auth_key......'; constructor(private http: HttpClient) { } public async AllCustomers(): Promise<ICustomer[]> { const apiUrl = `${this.baseUrl}/customers`; return this.http.get<ICustomer[]>(apiUrl , {headers: new HttpHeaders({Authorization: this.authKey})}).toPromise();<===== } }
When i replace the headers like this:
headers: new HttpHeaders({Authorization: this.authKey})
The default headers values(i,e Content-Type : application/json) will be replaced by the above headers.
Instead of replacing the headers how can i add
custom headers
, I tried like this:public async AllCustomers(): Promise<ICustomer[]> { const apiUrl = `${this.baseUrl}/courses`; const headers = new HttpHeaders(); headers.append('Authorization', this.authKey); headers.append('x-Flatten', 'true'); headers.append('Content-Type', 'application/json'); return this.http.get<ICustomer[]>(apiUrl).toPromise(); }
What's wrong with my approach, I am new to
angular
, Any help?-
CruelEngine almost 5 yearsIf you want to send auth token why don't you use an http interceptor ?
-
-
SGR almost 5 yearsI have added
header
to get request as you told. Got this errorERROR Error: Uncaught (in promise): HttpErrorResponse: {"headers":{"normalizedNames":{},"lazyUpdate":null,"headers":{}},"status":401,"statusText":"Unauthorized","url"
-
Tony Ngo almost 5 yearsThen your auth key is wrong. Check your auth key again