error NG8001: 'router-outlet' is not a known element
Solution 1
Here you are using RouterTestingModule
this is only use for unit try to use RouterModule
Like this
import { RouterModule } from '@angular/router';
@NgModule({
imports: [
RouterModule.forRoot(appRoutes)
],
...
})
Solution 2
I had the same problem because I was trying to import a Module which would declare a specific set of components. One of those components had a <router-outlet>
tag.
This was the error during the compilation:
ERROR in src/app/components/layout/layout.component.html:8:17 - error NG8001: 'router-oulet' is not a known element:
1. If 'router-oulet' is an Angular component, then verify that
it is part of this module.
2. If 'router-oulet' is a Web Component then add 'CUSTOM_ELEMENTS_SCHEMA' to the '@NgModule.schemas' of this component to suppress this message.
8 <router-oulet></router-oulet>
What I did was to import RouterModule on the external module, like this:
@NgModule({
declarations:[
AlertsComponent,
FooterComponent,
LogoutComponent,
MessageComponent,
SearchComponent,
SidebarComponent,
TopbarComponent,
UserInfoComponent,
LayoutComponent,
],
imports:[
RouterModule
]
})
export class LayoutModule {}
then I imported the module on the app.module.ts
@NgModule({
declarations: [
AppComponent
],
imports: [
AppRoutingModule,
BrowserModule,
LayoutModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Now it's working
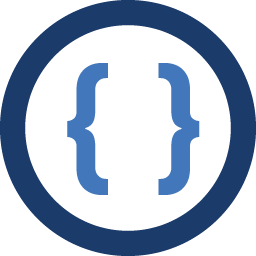
Admin
Updated on June 26, 2022Comments
-
Admin almost 2 years
error NG8001: 'router-outlet' is not a known element: 1. If 'router-outlet' is an Angular component, then verify that it is part of this module. 2. If 'router-outlet' is a Web Component then add 'CUSTOM_ELEMENTS_SCHEMA' to the '@NgModule.schemas' of this component to suppress this message.
4 <router-outlet></router-outlet>
In my app.component the line
<router-outlet></router-outlet>
In my package.json
{ "name": "auth-table2", "version": "0.0.0", "scripts": { "ng": "ng", "start": "ng serve", "build": "ng build", "test": "ng test", "lint": "ng lint", "e2e": "ng e2e" }, "private": true, "dependencies": { "@angular/animations": "~9.0.1", "@angular/common": "~9.0.1", "@angular/compiler": "~9.0.1", "@angular/core": "~9.0.1", "@angular/forms": "~9.0.1", "@angular/platform-browser": "~9.0.1", "@angular/platform-browser-dynamic": "~9.0.1", "@angular/router": "~9.0.1", "@ng-bootstrap/ng-bootstrap": "^6.0.0", "@ngrx/store": "^8.6.0", "angularfire2": "^5.4.2", "bootstrap": "^4.4.1", "firebase": "^7.9.1", "rxjs": "~6.5.4", "rxjs-compat": "^6.5.4", "tslib": "^1.10.0", "zone.js": "~0.10.2" }, "devDependencies": { "@angular-devkit/build-angular": "~0.900.2", "@angular/cli": "~9.0.2", "@angular/compiler-cli": "~9.0.1", "@angular/language-service": "~9.0.1", "@types/node": "^12.11.1", "@types/jasmine": "~3.5.0", "@types/jasminewd2": "~2.0.3", "codelyzer": "^5.1.2", "jasmine-core": "~3.5.0", "jasmine-spec-reporter": "~4.2.1", "karma": "~4.3.0", "karma-chrome-launcher": "~3.1.0", "karma-coverage-istanbul-reporter": "~2.1.0", "karma-jasmine": "~2.0.1", "karma-jasmine-html-reporter": "^1.4.2", "protractor": "~5.4.3", "ts-node": "~8.3.0", "tslint": "~5.18.0", "typescript": "~3.7.5" } }
In app.module.ts
import { AdminAuthGuard } from './admin-auth-guard.service'; import { UserService } from './user.service'; import { AuthGuard } from './auth-guard.service'; import { AuthService } from './auth.service'; import { environment } from './../environments/environment'; import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AngularFireModule } from 'angularfire2'; import { AngularFireDatabaseModule } from 'angularfire2/database'; import { AngularFireAuthModule } from 'angularfire2/auth'; import { RouterModule } from '@angular/router'; import { RouterTestingModule } from '@angular/router/testing'; import { NgbModule } from '@ng-bootstrap/ng-bootstrap'; import { AppComponent } from './app.component'; import { BsNavbarComponent } from './bs-navbar/bs-navbar.component'; import { HomeComponent } from './home/home.component'; import { ProductsComponent } from './products/products.component'; import { ShoppingCartComponent } from './shopping-cart/shopping-cart.component'; import { CheckOutComponent } from './check-out/check-out.component'; import { OrderSuccessComponent } from './order-success/order-success.component'; import { MyOrdersComponent } from './my-orders/my-orders.component'; import { AdminProductsComponent } from './admin/admin-products/admin-products.component'; import { AdminOrdersComponent } from './admin/admin-orders/admin-orders.component'; import { LoginComponent } from './login/login.component'; @NgModule({ declarations: [ AppComponent, BsNavbarComponent, HomeComponent, ProductsComponent, ShoppingCartComponent, CheckOutComponent, OrderSuccessComponent, MyOrdersComponent, AdminProductsComponent, AdminOrdersComponent, LoginComponent ], imports: [ BrowserModule, AngularFireModule.initializeApp(environment.firebase), AngularFireDatabaseModule, AngularFireAuthModule, // NgbModule.forRoot(), RouterTestingModule, RouterModule.forRoot([ { path: '', component: HomeComponent }, { path: 'products', component: ProductsComponent }, { path: 'shopping-cart', component: ShoppingCartComponent }, { path: 'login', component: LoginComponent }, { path: 'check-out', component: CheckOutComponent, canActivate: [AuthGuard] }, { path: 'order-success', component: OrderSuccessComponent, canActivate: [AuthGuard] }, { path: 'my/orders', component: MyOrdersComponent, canActivate: [AuthGuard] }, { path: 'admin/products', component: AdminProductsComponent, canActivate: [AuthGuard, AdminAuthGuard] }, { path: 'admin/orders', component: AdminOrdersComponent, canActivate: [AuthGuard, AdminAuthGuard] } ]) ], providers: [ AuthService, AuthGuard, AdminAuthGuard, UserService ], exports: [ [ RouterModule ] ], bootstrap: [AppComponent] }) export class AppModule { }