ASP MVC4 - Pass List to view via view model
Solution 1
You are using the same list_p
instance over and over again inside the loop. So you are constantly updating its Person property. And since Person
is a reference type you are modifying the same reference in memory. At the last iteration of the loop you are obviously replacing this reference with the last instance of Person which explains why you are seeing the same person in the view.
Try like this, seems lot easier:
public ActionResult PersonList()
{
ViewBag.Message = "My List";
var model = db.Person.Select(p => new vm_PersonList
{
Person = p,
age = 23
}).ToList();
return View(model);
}
Solution 2
You are working on the same instance of vm_PersonList. Move the instantiation of vm_PersonList into the loop
foreach (var p in db.Person)
{
var list_p = new vm_PersonList();
list_p.Person = p;
//the age will be calculated based on p.birthDay, not relevant for the
//current question
list_p.age = 23;
list.Add(list_p);
}
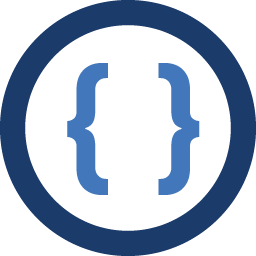
Admin
Updated on May 26, 2020Comments
-
Admin almost 4 years
I have a model Person (with among other fields the day of Birth) and I want to pass a list of all persons, together with the calculated age of each person, to the view
Therefor:
The view model
public class vm_PersonList { public Person Person { get; set; } public int age { get; set; } }
The controller action:
public ActionResult PersonList() { ViewBag.Message = "My List"; var list = new List<vm_PersonList>(); var list_p = new vm_PersonList(); foreach (var p in db.Person) { list_p.Person = p; //the age will be calculated based on p.birthDay, not relevant for the //current question list_p.age = 23; list.Add(list_p); } return View(list); }
The view
@model List<programname.Viewmodels.vm_PersonList> @foreach (var p in Model) { <tr> <td> @p.Person.FullName </td> <td> @p.age </td> </tr> }
The Person table contains for example 6 entries. When debugging the application I see:
At the end of the controller action "list" contains correctly the 6 different Person entries
In the view, the "Model" contains 6 entries, but 6 times the last "database entry". Does anyone have a suggestion to solve this issue?