Assembly x86 putting values into array with loop
myArray = 0, 1, 2, 3, 4, 5, 6, 7, 8, 9.
These are the numbers that you want your array to contain. But since you initialized the index variable (that you will be using for both indexing and storing) as 1 (using index db 1
) this will lead to another result.
Just setup the index with:
index db 0
There's another reason to set it up this way!
In the notation [intArray+index]
the index
part is meant to be an offset in the array. Offsets are always zero based quantities. The way you wrote your program, it will write the 10th value behind the array.
add [intArray+index], al ; here is the problem
You're right that this is the problem. Some assemblers won't compile this, and others will just add the addresses of both these variables. Neither suits your purpose. What you need is putting the content of the index variable in a register and use that combination of operands.
intArray db 10 dup (0)
index db 0
...
loopArray:
movzx bx, [index]
mov [intArray+bx], bl ;Give the BX-th array element the value BL
inc [index]
cmp [index], 10
jb loopArray
With this code the index will start at 0 and then the loop will continu for as long as the index is smaller than 10.
Of course you could write this program without using an index variable at all.
intArray db 10 dup (0)
...
xor bx, bx ;This make the 'index' = 0
loopArray:
mov [intArray+bx], bl ;Give the BX-th array element the value BL
inc bx
cmp bx, 10
jb loopArray
Given that the array was initially filled with zeroes, you can replace the:
mov [intArray+bx], bl ;Give the BX-th array element the value BL
with:
add [intArray+bx], bl ;Give the BX-th array element the value BL
Do remember this can only work if the array was filled with zeroes beforehand!
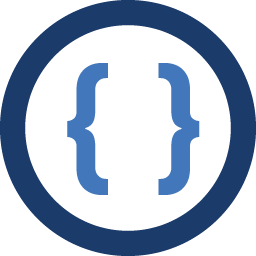
Admin
Updated on May 30, 2020Comments
-
Admin almost 4 years
I wanted to put numbers in an array of length 10, but each number is 1 bigger than the last number. It means:
myArray = 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
I have tried this:
IDEAL
MODEL small
STACK 100hDATASEG
intArray db 10 dup (0)
index db 1CODESEG
start:
mov ax, @DATA
mov ds, ax
loopArray:
mov al, [index]
add [intArray+index], al ; here is the problem
inc [index]
cmp [index], 11
jb loopArray
exit:
mov ax, 4c00h
int 21h
END startBut I can't add the index to [intArray + index], so I tried to to add it to [intArray+al], and that doesn't work either.
How can I add the index to the next array's value each time?