Bubble sort on array on Assembly Language
Solution 1
For the 1st error you forgot to type a comma between the register and the immediate.
For the 2nd and 3rd errors the CH and CL registers cannot be used for addressing memory. Use SI, DI, or BX instead.
Since your array is defined as words you must treat it as such!
Change
mov dh,[ARR+ch]
mov dl,[ARR+cl]
into something like (depends on other choices you make)
mov ax,[ARR+si]
mov dx,[ARR+di]
Please note that you placed the array amidst the instructions. This will crash your program as soon as you manage to compile it. Either place the array in a separate data segment of your program or jump over this line.
start:
mov ax,code
mov ds,ax
jmp start2
ARR: dw 1,2,4,3,6,5,9
start2:
mov ch,0h
Solution 2
This is simple code to bubble sort
iclude'emu8086.inc'
org 100h
.data
array db 9,6,5,4,3,2,1
count dw 7
.code
mov cx,count
dec cx ; outer loop iteration count
nextscan: ; do { // outer loop
mov bx,cx
mov si,0
nextcomp:
mov al,array[si]
mov dl,array[si+1]
cmp al,dl
jnc noswap
mov array[si],dl
mov array[si+1],al
noswap:
inc si
dec bx
jnz nextcomp
loop nextscan ; } while(--cx);
;;; this loop to display elements on the screen
mov cx,7
mov si,0
print:
Mov al,array[si]
Add al,30h
Mov ah,0eh
Int 10h
MOV AH,2
Mov DL , ' '
INT 21H
inc si
Loop print
ret
Solution 3
; SORTING ARRAY BY USING BUBBLE SORT ALGORITHM
.MODEL SMALL
.STACK 100H
.DATA
N DB 44H,22H,11H,55H,33H ; N is an array
LEN DW 5 ; LENGTH OF ARRAY N
.CODE
MAIN PROC
MOV AX,@DATA
MOV DS,AX
MOV CX,LEN ;Cx is counter for OUTERLOOP CX=5
DEC CX ; CX = 4
OUTERLOOP:
MOV SI,0 ; SI is the index of array N
MOV DX,CX ; Dx is counter for INNERLOOP
INNERLOOP:
MOV AH,N[SI] ; assign the number N[SI] into reg.AH
MOV AL,N[SI+1] ; assign the next number N[SI+1] into reg.AL
CMP AH,AL ; Compare between N[SI] and N[SI+1] <BR>
JC CARRY ; if AL > AH => Carry Flag =1 ,THEN jump to carry
MOV N[SI] , AL ; else , Do Switching bteween N[SI] and N[SI+1]
MOV N[SI+1] ,AH
CARRY:
INC SI
DEC DX
JNZ INNERLOOP
LOOP OUTERLOOP
;exit
MOV AH,4CH ;service number
INT 21H ; interrupt
MAIN ENDP
END
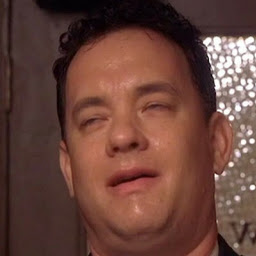
Puloko
Updated on April 09, 2020Comments
-
Puloko about 4 years
I need to Bubblesort an unorganized array with 7 integers from biggest to smallest so it would look like 9,6,5,4,3,2,1.
I ran my code through the compiler and it saysI can't understand what is the problem with this code:
code segment assume ds:code,cs:code start: mov ax,code mov ds,ax ;code start ARR: dw 1,2,4,3,6,5,9 mov ch,0h mov cl,1h mov bh 7h jmp assign_nums restart: mov ch,0h mov cl,1h dec bh jmp assign_nums swap: mov ch,dl mov cl,dh jmp next next: cmp bh,cl je restart add ch,1h add cl,1h jmp assign_nums assign_nums: cmp bh,0h je done mov dh,[ARR+ch] mov dl,[ARR+cl] cmp dh,dl jl swap jnl next done: nop code ends end start