AttributeError: 'Sequential' object has no attribute 'output_names'
Solution 1
As @Shinva said to set the "compile" attribute of the load_model function to "False". Then after loading the model, compile it separately.
from tensorflow.keras.models import save_model, load_model
save_model(model,'124446.model')
Then for loading the model again do:
saved_model = load_model('124446.model', compile=False)
saved_model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
saved_model.predict([x_test])
Update: For some unknown reasons, I started to get the same errors as the question states. After trying to find different solutions it seems using the "keras" library directly instead of "tensorflow.keras" works properly.
My setup is on "Windows 10" with python:'3.6.7', tensorflow:'1.11.0' and keras:'2.2.4'
As per my knowledge, there are three different ways in which you can save and restore your model; provided you have used keras directly to make your model.
Option1:
import json
from keras.models import model_from_json, load_model
# Save Weights + Architecture
model.save_weights('model_weights.h5')
with open('model_architecture.json', 'w') as f:
f.write(model.to_json())
# Load Weights + Architecture
with open('model_architecture.json', 'r') as f:
new_model = model_from_json(f.read())
new_model.load_weights('model_weights.h5')
Option2:
from keras.models import save_model, load_model
# Creates a HDF5 file 'my_model.h5'
save_model(model, 'my_model.h5') # model, [path + "/"] name of model
# Deletes the existing model
del model
# Returns a compiled model identical to the previous one
new_model = load_model('my_model.h5')
Option 3
# using model's methods
model.save("my_model.h5")
# deletes the existing model
del model
# load the saved model back
new_model = load_model('my_model.h5')
Option 1 requires the new_model to be compiled before using.
Option 2 and 3 are almost similar in syntax.
Codes used from:
1. Saving & Loading Keras Models
2. https://keras.io/getting-started/faq/#how-can-i-save-a-keras-model
Solution 2
I was able to load the model by setting compile=False in load_model()
Solution 3
import tensorflow as tf
tf.keras.models.save_model(
model,
"epic_num_reader.model",
overwrite=True,
include_optimizer=True
)
new_model = tf.keras.models.load_model('epic_num_reader.model', custom_objects=None, compile=False)
predictions = new_model.predict(x_test)
print(predictions)
import numpy as np
print(np.argmax(predictions[0]))
plt.imshow(x_test[0],cmap=plt.cm.binary)
plt.show()
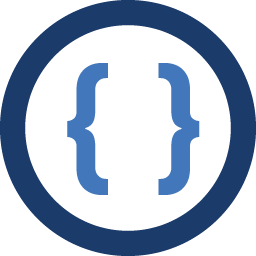
Admin
Updated on July 07, 2022Comments
-
Admin almost 2 years
I have got some problem for the below code of the following line new_model = load_model('124446.model', custom_objects=None, compile=True) Here is the code:
import tensorflow as tf from tensorflow.keras.models import load_model mnist = tf.keras.datasets.mnist (x_train,y_train), (x_test,y_test) = mnist.load_data() x_train = tf.keras.utils.normalize(x_train,axis=1) x_test = tf.keras.utils.normalize(x_test,axis=1) model = tf.keras.models.Sequential() model.add(tf.keras.layers.Flatten()) model.add(tf.keras.layers.Dense(128,activation=tf.nn.relu)) model.add(tf.keras.layers.Dense(128,activation=tf.nn.relu)) model.add(tf.keras.layers.Dense(10,activation=tf.nn.softmax)) model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy']) model.fit(x_train,y_train,epochs=3) tf.keras.models.save_model(model,'124446.model') val_loss, val_acc = model.evaluate(x_test,y_test) print(val_loss, val_acc) new_model = load_model('124446.model', custom_objects=None, compile=True) prediction = new_model.predict([x_test]) print(prediction)
Errors are:
Traceback (most recent call last): File "C:/Users/TanveerIslam/PycharmProjects/DeepLearningPractice/1.py", line 32, in new_model = load_model('124446.model', custom_objects=None, compile=True) File "C:\Users\TanveerIslam\PycharmProjects\DeepLearningPractice\venv\lib\site-packages\tensorflow\python\keras\engine\saving.py", line 262, in load_model sample_weight_mode=sample_weight_mode) File "C:\Users\TanveerIslam\PycharmProjects\DeepLearningPractice\venv\lib\site-packages\tensorflow\python\training\checkpointable\base.py", line 426, in _method_wrapper method(self, *args, **kwargs) File "C:\Users\TanveerIslam\PycharmProjects\DeepLearningPractice\venv\lib\site-packages\tensorflow\python\keras\engine\training.py", line 525, in compile metrics, self.output_names)
AttributeError: 'Sequential' object has no attribute 'output_names'
So can any one give me ant solution.
Note: I use pycharm as IDE.
-
Suraj Rao almost 5 yearsWhile this code may solve the question, including an explanation of how and why this solves the problem would really help to improve the quality of your post, and probably result in more up-votes. Remember that you are answering the question for readers in the future, not just the person asking now. Please edit your answer to add explanations and give an indication of what limitations and assumptions apply.