AttributeError list object has no attribute add
the message is clear. list
has no method add
because it is ordered (it has a dunder __add__
method but that's for addition between lists). You can insert
but you want to append
. So the correct way is:
X_train = []
for row in cur:
X_train.append(row)
BUT the preferred way converting to a list directly (iterating on cur
elements to create your list in a simple and performant way):
X_train = list(cur)
BUT you cannot do that since your list contains bogus data. Fortunately, you can filter them out in a nested list comprehension like this:
X_train = [[x for x in r if type(x)==int] for r in cur]
this builds your list of lists but filters out non-integer values and feeding it to numpy.asarray
yields (with your sample data):
[[ 6 1 1 1 2 1 0 0 19]
[ 6 1 1 1 2 1 0 0 14]]
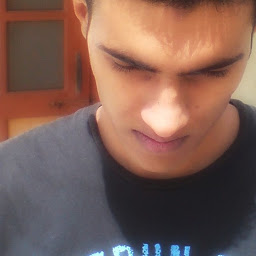
Abbas Zahid
Updated on December 21, 2020Comments
-
Abbas Zahid over 3 years
Python is new for me and I am doing some machine learning code using python. My scenario is that I am reading data from my sql and trying to give a shape to this data so i can use it for MLP training.
My code is below:
connection = mysql.connector.connect(host='localhost', port=3306, user='root', passwd='mysql', db='medicalgame') cur = connection.cursor() query = "" cur.execute(query) # X_train will be a list of list and later we'll convert it to a numpy ndarray X_train = [] for row in cur: X_train.add(row) connection.close() X_train should be ready X_train = np.asarray(X_train) print 'The shape of X_train is', X_train.shape
During debug the query result i got is like this: (6, 1, 1, 1, 2, u'F', 1, 0, 0, 19) Can anyone help me how can, I fix the error and give shape to my X_train, so that MLP accept it as an input ?