Bad state: field does not exist within the DocumentSnapshotPlatform . It shows the getter was called on null
Solution 1
Edit
Now that I've become little better than before, so I must update this answer for better understanding.
Let's take this above example:
return ListView.builder(
reverse: true,
itemCount: documents.length,
itemBuilder: (context, index) {
return MessageBubble(
documents[index]['text'],
documents[index]['userID'] == user.uid,
documents[index]['userID'],
documents[index]['imageurl']);
},
);
},
);
Here, all the documents in that particular collection MUST contain these 3 fields, i.e, 'text'
, 'userID'
, and 'imageurl'
to successfully build each child inside the listView.builder()
.
But, if any of these 3 fields is missing, or even miss-spelled in One or more documents, then it would throw an error like the above.
Will throw error:
Will throw error:
So, to solve that we need to find that particular document which has the field missing, or field miss-spelled and correct it.
Or, delete that document and keep only those documents which has correct fields.
(By the way, I'm using "Dark Reader" chrome extension for dark theme for firebase console, and every other website on internet.)
My Old Answer:
In my case I was using Firebase Firestore for my chat app, and while loading the chat screen, I was getting this error.
So in Firestore Database I had 15-20 messages, So I deleted most of them and leave just 2-3 messages. And then the Error disappeared.
I don't know happened here.
Solution 2
Try the following:
if (snapshot.connectionState == ConnectionState.waiting)
Center(child: CircularProgressIndicator());
else if(snapshot.connectionState == ConnectionState.active){
final documents = snapshot.data.documents;
return ListView.builder(
reverse: true,
itemCount: docs.length,
itemBuilder: (context, index) {
return MessageBubble(
docs[index]['text'],
docs[index]['userID'] == user.uid,
docs[index]['userID'],
docs[index]['imageurl']);
},
);
},
},
);
When the connectionState is active meaning with data being non-null, then retrieve the data. Also use docs
since it seems you are using the new version of Firestore.
documents
became docs
Solution 3
You create a widget and then don't use it and follow through with the rest of your logic:
if (snapshot.connectionState == ConnectionState.waiting)
Center(child: CircularProgressIndicator());
You need to stop there and return that widget from your builder:
if (snapshot.connectionState == ConnectionState.waiting)
return Center(child: CircularProgressIndicator());
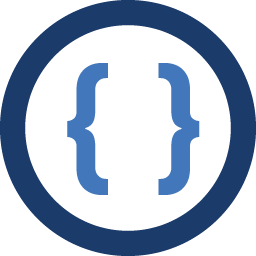
Admin
Updated on December 26, 2022Comments
-
Admin over 1 year
The relevant error-causing widget was StreamBuilder. I've given the link of the project repo in github .
Widget build(BuildContext context) { return StreamBuilder( stream: FirebaseFirestore.instance .collection("/chats/2DGQmBssJ4sU6MVJZYc0/messages") .orderBy('createdAt', descending: true) .snapshots(), builder: (context, snapshot) { if (snapshot.connectionState == ConnectionState.waiting) Center(child: CircularProgressIndicator()); final documents = snapshot.data.documents; return ListView.builder( reverse: true, itemCount: documents.length, itemBuilder: (context, index) { return MessageBubble( documents[index]['text'], documents[index]['userID'] == user.uid, documents[index]['userID'], documents[index]['imageurl']); }, ); }, );
} }
-
Admin over 3 yearsi tried but now it just a blank white screen. The progress indicator is wroking but when the connection is active .It doesn't return the content of the build by the StreamBuilder
-
Admin over 3 yearsWhat should i return at the end? Because needs an widget in return .
-
Peter Haddad over 3 years
return Center(child: CircularProgressIndicator());