MissingPluginException(No implementation found for method Firebase#initializeCore on channel plugins.flutter.io/firebase_core) on Android
Solution 1
Setting minifyEnabled & shrinkResources to false fixes it but why? I do not know. If somebody know please write a comment.
buildTypes {
release {
signingConfig signingConfigs.release
minifyEnabled false
shrinkResources false
}
}
Solution 2
Recently Added Firebase
Depending on how your project is structured adding any new Firebase package might require a complete reset of the app on the device (deleting initial values):
- Uninstall the app from the device
- Close and reopen Android Studio (sometimes optional)
- Run/Start the application again
Done!
Solution 3
What I ended up realizing after running into this issue, is that the error is super vague and doesn't necessarily mean there's a problem with firebase_core
, rather there could be an issue with any of the Firebase plugins. So to narrow down where the issue was, I went through each firebase import individually and commented it out in the Pubspec.yaml file and ran it (along with commenting out all the code in my project that used that import), until I found which package was causing the issue.
For me, I was experiencing the issue only when running on iOS. It turned out that the firebase_messaging
was the culprit and it was code that I had added to the iOS AppDelegate to handle the messaging that was no longer needed with the latest version of the firebase_messaging
plugin.
Solution 4
It's difficult to say exactly what's wrong without sifting through your entire project, but here are a few actions that will likely remedy the problem.
- Run
flutter doctor
and verify everything is working as expected. - Run
flutter clean
followed byflutter pub get
.
If you're still receiving the same error, then you likely made a mistake while editing one of the config files.
- Compare your android/app/main/AndroidManifest.xml to the previous working version (specifically ensure you didn't accidentally delete any
<meta-data />
tags as doing so would result in said error. - Do the same with the android/build.gradle and android/app/build.gradle.
Solution 5
I started a fresh project and made sure to change:
classpath 'com.android.tools.build:gradle:4.2.0-alpha07'
to the version officially supported: 3.5.0
Not sure if that downgrade was necessary because as I say, I restarted an entirely fresh project and something else may have effected it.
I also filed a ticket with Google which may prove helpful for anyone else in the future: https://github.com/FirebaseExtended/flutterfire/issues/3212#issuecomment-675407420
Related videos on Youtube
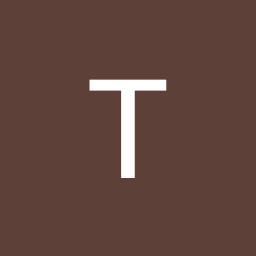
Tom Taila
Updated on July 09, 2022Comments
-
Tom Taila almost 2 years
I am getting the above error when calling
Firebase.initializeApp()
in my flutter code. I have followed the documentation here: https://firebase.flutter.dev/docsHere is my pubspec.yaml
dependencies: flutter: sdk: flutter firebase_core: ^0.5.0 cloud_firestore: ^0.14.0 firebase_auth: ^0.18.0 fl_chart: ^0.11.0 snapping_sheet: ^2.0.0 flutter_svg: ^0.18.0 flutter_redux: ^0.6.0 strings: ^0.1.2 random_string: ^2.1.0 redux_thunk: ^0.3.0 # firebase_crashlytics: ^0.1.4+1 dotted_line: ^2.0.1 # The following adds the Cupertino Icons font to your application. # Use with the CupertinoIcons class for iOS style icons. cupertino_icons: ^0.1.3 dev_dependencies: flutter_test: sdk: flutter
Here is my flutter code:
void main() { WidgetsFlutterBinding.ensureInitialized(); SystemChrome.setEnabledSystemUIOverlays(SystemUiOverlay.values); final store = Store<AppState>(AppState.reducer, initialState: AppState.initial(), middleware: [thunkMiddleware]); runApp( FutureBuilder( future: Firebase.initializeApp(), builder: (context, snapshot) { // Check for errors if (snapshot.hasError) { log(snapshot.error.toString()); return Container(color: Colors.red); } // Once complete, show your application if (snapshot.connectionState == ConnectionState.done) { return StoreProvider<AppState>( store: store, child: MoollaApp(store: store), ); } // Otherwise, show something whilst waiting for initialization to complete return Container(color: Colors.green); }, ), ); }
Here are the relevant part of my .gradle (app) file:
plugins { id "com.android.application" id "com.google.gms.google-services" id "kotlin-android" id "kotlin-android-extensions" }
Here is my project gradle file:
// Top-level build file where you can add configuration options common to all sub-projects/modules. buildscript { ext.kotlin_version = "1.3.72" repositories { google() jcenter() mavenCentral() } dependencies { classpath 'com.android.tools.build:gradle:4.2.0-alpha07' classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version" classpath 'com.google.gms:google-services:4.3.3' } } allprojects { repositories { google() jcenter() mavenCentral() } } rootProject.buildDir = '../build' subprojects { project.buildDir = "${rootProject.buildDir}/${project.name}" } subprojects { project.evaluationDependsOn(':app') } task clean(type: Delete) { delete rootProject.buildDir }
and my Application class extension:
class MyApp : FlutterApplication() { override fun onCreate() { super.onCreate() } }
As far as I can tell I have done everything correctly according to the docs.
-
John Alexander Betts over 3 years
cloud_firestore
0.14.0 has been released yesterday. I have the same issue -
Taslim over 3 yearsI know this comment is too late. Check your ChannelMethod("") you may used anywhere in your code. You should not use everywhere channel name as package name.
-
-
Jeff S. about 3 yearsIn my case, it was the flutter_apns plugin and the problem only occurred on iOS. I've then noticed that it provides the specific flag "flutter_apns.disable_firebase_core" (mentioned in the readme) to enable the parallel use of firebase core.
-
Simon Hutton over 2 yearsThis saved my bacon big style. I had released a production upgrade having set
minifyEnabled true
(amongst other things) which resulted in a black screen ANR. Changing it back tofalse
fixed this issue. Cheers Mehmet -
Vatsal Dholakiya over 2 yearsThis does not provide an answer to the question. Once you have sufficient reputation you will be able to comment on any post; instead, provide answers that don't require clarification from the asker.
-
madhairsilence over 2 yearsOk. This is in deed a valuable answer. If you are adding firebase code to already installed app, you need to delete and reinstall it
-
Migalv over 2 yearsInsane. Thanks a lo @Mehmet you saved my life. I've been having this issue for 4 days now and it was driving me crazy!
-
nelsonspbr about 2 yearsThanks, this is exactly what I needed.
-
Jay Shenawy almost 2 yearsThis solved my problem thank you , in build.gradle file , make sure you use a version no earlier than classpath 'com.android.tools.build:gradle:4.1.0'