Best practice to convert list object to list objectDto java
11,758
Instead of re-inventing the wheel and writing this by yourself you better use an existing library/tool, which is made for this.
I would recommend to use ModelMapper which is a great library for DTO/entity mapping, you will just need one line to convert your entity to DTO, something like:
ModelMapper modelMapper = new ModelMapper();
BookDto bookDTO = modelMapper.map(book, BookDto.class);
You can check the library's Examples page or the Entity To DTO Conversion for a Spring REST API tutorial to get deeper into this library.
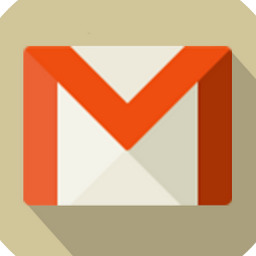
Author by
Akza
Updated on June 05, 2022Comments
-
Akza about 2 years
I have done do get all my data and convert from object to objectDto. But, somehow, I feel like my code is not good yet. Here I need your help, I need any reference/advice to make my code better (in performance). Here is my working code:
@Override public List<BookDto> findAll() throws Exception { try { List<Book> list = bookDao.findAll(); List<BookDto> listDto = new ArrayList<>(); for (Book book : list) { BookDto bookDto = new BookDto(); Set<AuthorDto> listAuthorDto = new HashSet<AuthorDto>(); Set<Author> dataAuthor = new HashSet<Author>(); book.getAuthor().iterator().forEachRemaining(dataAuthor::add); BeanUtils.copyProperties(book, bookDto, "author", "category"); bookDto.setCategory(book.getCategory().getCategory()); for (Author author : dataAuthor) { AuthorDto authorDto = new AuthorDto(); BeanUtils.copyProperties(author, authorDto); listAuthorDto.add(authorDto); } bookDto.setAuthor(listAuthorDto); listDto.add(bookDto); } return listDto; } catch (Exception e) { throw new Exception(e); } }
and here is the output that I need (already achieved with above code):
[ { "title": "book1", "year": "2013", "author": [ { "name": "john", "address": "NY" }, { "name": "angel", "address": "LA" } ], "category": "science" }, { "title": "book2", "year": "2014", "author": [ { "name": "john", "address": "NY" } ], "category": "science" }, { "title": "book3", "year": "2009", "author": [ { "name": "angel", "address": "LA" } ], "category": "comedy" } ]
-
sklott almost 5 yearsProbably using some existing library, like e.g. "Gson", will eliminate any need in your custom code at all.
-
Abhijeet Farakate almost 5 yearsYou can use a library like model mapper.
-
-
Dzmitry Prakapenka almost 5 yearsI prefer mapstruct, for the possibility to see the result of code generation after mapping and code post-processing.
-
Akza almost 5 yearswhy it is ridiculous? I thought it might be useful to trace error...?
-
cнŝdk almost 5 yearsWell they are several ways of doing it, we just need to choose the suitable one ;)