Bind JSON object to HTML element
Solution 1
If you'll be doing this a lot in your application, you may want to bring in a library such as the excellent Knockout.js which uses MVVM to do bindings as you describe.
Your markup would look something like this:
<div data-bind="text: firstName"></div>
<div data-bind="text: lastName"></div>
And in your JavaScript:
function MyViewModel() {
this.firstName = "John";
this.lastName = "Smith";
}
ko.applyBindings(new MyViewModel());
You can also use a data set if there are many "people." Try out this tutorial if you would like to learn how to do that: Working with Lists and Collections.
Other useful links:
Solution 2
First, add id
attributes to your markup (you could do this with the DOM but for clarity's sake id
s are probably best suited for this example):
<div class="person-list-item">
<div id="firstname">John</div>
<div id="lastname">Smith</div>
</div>
Use a jQuery event handler to update the fields whenever they are modified (this is an inefficient solution but gets the job done- worry about optimizing once you have something functional):
// declare your object
function person(firstn, lastn) {
this.firstname = firstn;
this.lastname = lastn;
}
var myGuy = new person("John", "Smith");
$(document).ready(function () {
$("#firstname").change(function () {
myGuy.firstname = $("#firstname").val();
});
$("#lastname").change(function () {
myGuy.lastname = $("#lastname").val();
});
// etc...
});
Now every time the fields are updated your object will be too.
Solution 3
Simple one-way approach using jquery...
var resp = your_json_data;
$(function () {
$(".jsondata").each(function () {
$(this).html(eval($(this).attr("data")));
});
});
then in the page...
<span class="jsondata" data="resp.result.formatted_phone_number" />
Solution 4
You can parse the JSON to turn it into a JavaScript object:
var data = $.parseJSON(json_string);
// or without jQuery:
var data = JSON.parse(json_string);
If I understood your question correctly, you should have JSON that look like this:
[
{
"firstName": "John",
"lastName": "Smith"
},
{
"firstName": "Another",
"lastName": "Person"
} // etc
]
So you can just loop through the people like so:
$(document).ready(function() {
var str = '[{"firstName":"John","lastName":"Smith"},{"firstName":"Another","lastName": "Person"}]',
data = $.parseJSON(str);
$.each(data, function() {
var html = '<div class="person-list-item"><div>' + this.firstName + '</div><div>' + this.lastName + '</div></div>';
$('#person-list').append(html);
});
});
Fiddle: http://jsfiddle.net/RgdhX/2/
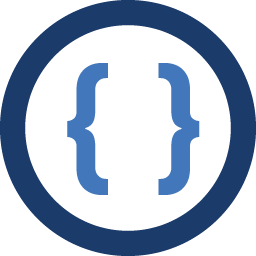
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I want to bind a JSON object to a HTML element.
e.g.
I have a object "person" with the attributes "firstName", "lastName"
<div class="person-list"> <div class="person-list-item"> <div>John</div> ---> bind it to person.firstName <div>Smith</div> ---> bind it to person.lastName </div> </div>
so, if a value of the HTML elements gets changed, then will also the object person gets updated.
is this possible in any way?
i use:
- jquery
- ASP.NET MVC 3