How to manipulate HTML in Flutter webview?
860
So, it depends what library you are using to load your webview.
I have had some good success using webview_flutter_plus
(https://pub.dev/packages/webview_flutter_plus).
With this, you just need to wait for your html to load and inject some javascript to modify whatever html you want.
Like this:
Widget build(BuildContext context) {
return WebViewPlus(
initialUrl: myUrl,
javascriptMode: JavascriptMode.unrestricted,
onWebViewCreated: (WebViewPlusController webViewController) {
_webViewController = webViewController;
},
onPageFinished: (String url) {
_webViewController.evaluateJavascript('document.querySelector(".name").innerHTML = "new text";');
}
}
}
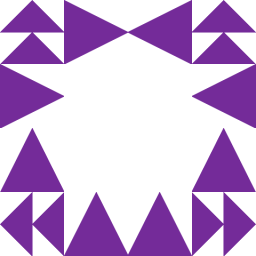
Author by
FlutterFirebase
Updated on December 24, 2022Comments
-
FlutterFirebase 11 months
I am load website with Flutter webview. But I want replace HTML element when it load. For example:
<span class="name">Replace text</span>
How to do?
-
IronMan about 3 yearsHi, you could attach a handler to the load event
document.addEventListener("DOMContentLoaded", function() { // do my replacement });
-
FlutterFirebase about 3 years@IronMan How to do with Flutter webview?
-
-
kikicoder over 2 yearsdear @Didier Prophete I using this way but it seems evaluating jave script takes too long what I could done about that
-
Didier Prophete over 2 yearsYeah. The truth is that binging up a webview inside a native app in flutter does take some time (like half a second). After that I think the js evaluation is fine but that initial time is a giant pain. Not sure there is much we can do there until flutter itself improves the webview perf (and there as well, I am not sure flutter can do anything. It might just be that a webview is an expensive class to instanciate...)
-
kikicoder over 2 yearsDear is there a way I could manage to not showing the webview until it evaluate, I mean the evaluation happens on onpagefinished callback and that it self need some time to be fired
-
Didier Prophete over 2 yearsyes @kikicoder, that's exactly what I do in my own code. I basically hide the webview (I put it in a stack, below a splash page) until onPageFinished is invoked...