Black Screen in flutter when navigated to different page using gesturedetector in flutter. Says multiple heros share same tag
Solution 1
The error multiple heroes on one route can't have the same tag
is possible when you try to use multiple FloatingActionButtons
in one widget.
According to the documentation of heroTag
in the FloatingActionButton
:
The tag to apply to the button's Hero widget.
Defaults to a tag that matches other floating action buttons.
Set this to null explicitly if you don't want the floating action button to have a hero tag.
If this is not explicitly set, then there can only be one FloatingActionButton per route (that is, per screen), since otherwise there would be a tag conflict (multiple heroes on one route can't have the same tag). The material design specification recommends only using one floating action button per screen.
To resolve your exception and Use multiple FAB in a single widget make sure to pass Unique
object/value/string for heroTag
in the FAB.
Note: If you don't pass a value to heroTag
it creates a default value <default FloatingActionButton tag>
. which is common for all FABs. So if they are in the same widget they'll be using duplicate heroTag
which throws exception.
Let me know if you have any doubts.
Solution 2
It might be late but, if you still confusing. I solved my problem by manually added new attribute heroTag
in my FloatingActionButtons.
@override
Widget build(BuildContext context) {
return Row(
mainAxisAlignment: MainAxisAlignment.end,
children: <Widget>[
FloatingActionButton(
heroTag: 1, // put something different with this
onPressed: (){
counterBloc.add(CounterEvent.increment);
},
child: Icon(Icons.add),
),
FloatingActionButton(
heroTag: 2, // put something different with this
onPressed: (){
counterBloc.add(CounterEvent.decrement);
},
child: Icon(Icons.remove),
),
],
);
}
Hope it's Help someone
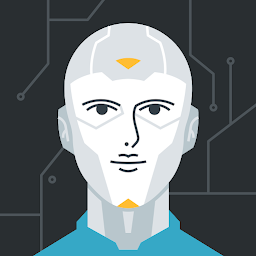
Jithin Palepu
Updated on December 16, 2022Comments
-
Jithin Palepu over 1 year
I am building an application where Home ui looks like grid and when tapped on an individual tile of the grid user will be navigated to another page. But whenever it happens an error throws up. Here is the code not looking at syntactical errors. I did not use any hero widget. Though i get this error..
body: Container( child: GridView.count( crossAxisCount: 2, children: <Widget>[ Card( child: InkWell( onTap: (){ print('tapped pnemonia'); Navigator.push(context, MaterialPageRoute(builder: (context)=> diagnosis())); }, child: Center( child: Column( children: <Widget>[ Text('Pnemonia'), Image.asset('assets/images/lungs.jpg'), ], ), ), ), ), ], ), ), ); } }
and the output error is the following:
I/flutter ( 6827): ├# Here is the subtree for one of the offending heroes: Hero I/flutter ( 6827): I/flutter ( 6827): When the exception was thrown, this was the stack: I/flutter ( 6827): #0 Hero._allHeroesFor.inviteHero.<anonymous closure> (package:flutter/src/widgets/heroes.dart:265:11) I/flutter ( 6827): #1 Hero._allHeroesFor.inviteHero (package:flutter/src/widgets/heroes.dart:276:8) I/flutter ( 6827): #2 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:295:21) I/flutter ( 6827): #3 SingleChildRenderObjectElement.visitChildren (package:flutter/src/widgets/framework.dart:5433:14) I/flutter ( 6827): #4 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #5 ComponentElement.visitChildren (package:flutter/src/widgets/framework.dart:4272:14) I/flutter ( 6827): #6 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #7 SingleChildRenderObjectElement.visitChildren (package:flutter/src/widgets/framework.dart:5433:14) I/flutter ( 6827): #8 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #9 MultiChildRenderObjectElement.visitChildren (package:flutter/src/widgets/framework.dart:5534:16) I/flutter ( 6827): #10 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #11 SingleChildRenderObjectElement.visitChildren (package:flutter/src/widgets/framework.dart:5433:14) I/flutter ( 6827): #12 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #13 ComponentElement.visitChildren (package:flutter/src/widgets/framework.dart:4272:14) I/flutter ( 6827): #14 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #15 SingleChildRenderObjectElement.visitChildren (package:flutter/src/widgets/framework.dart:5433:14) I/flutter ( 6827): #16 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #17 ComponentElement.visitChildren (package:flutter/src/widgets/framework.dart:4272:14) I/flutter ( 6827): #18 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #19 MultiChildRenderObjectElement.visitChildren (package:flutter/src/widgets/framework.dart:5534:16) I/flutter ( 6827): #20 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #21 ComponentElement.visitChildren (package:flutter/src/widgets/framework.dart:4272:14) I/flutter ( 6827): #22 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #23 ComponentElement.visitChildren (package:flutter/src/widgets/framework.dart:4272:14) I/flutter ( 6827): #24 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #25 ComponentElement.visitChildren (package:flutter/src/widgets/framework.dart:4272:14) I/flutter ( 6827): #26 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #27 MultiChildRenderObjectElement.visitChildren (package:flutter/src/widgets/framework.dart:5534:16) I/flutter ( 6827): #28 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #29 ComponentElement.visitChildren (package:flutter/src/widgets/framework.dart:4272:14) I/flutter ( 6827): #30 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #31 ComponentElement.visitChildren (package:flutter/src/widgets/framework.dart:4272:14) I/flutter ( 6827): #32 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #33 ComponentElement.visitChildren (package:flutter/src/widgets/framework.dart:4272:14) I/flutter ( 6827): #34 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #35 SingleChildRenderObjectElement.visitChildren (package:flutter/src/widgets/framework.dart:5433:14) I/flutter ( 6827): #36 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #37 ComponentElement.visitChildren (package:flutter/src/widgets/framework.dart:4272:14) I/flutter ( 6827): #38 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #39 SingleChildRenderObjectElement.visitChildren (package:flutter/src/widgets/framework.dart:5433:14) I/flutter ( 6827): #40 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #41 ComponentElement.visitChildren (package:flutter/src/widgets/framework.dart:4272:14) I/flutter ( 6827): #42 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #43 ComponentElement.visitChildren (package:flutter/src/widgets/framework.dart:4272:14) I/flutter ( 6827): #44 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #45 ComponentElement.visitChildren (package:flutter/src/widgets/framework.dart:4272:14) I/flutter ( 6827): #46 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): #47 ComponentElement.visitChildren (package:flutter/src/widgets/framework.dart:4272:14) I/flutter ( 6827): #48 Hero._allHeroesFor.visitor (package:flutter/src/widgets/heroes.dart:308:15) I/flutter ( 6827): (elided 3 frames from package dart:async) I/flutter ( 6827): I/flutter ( 6827): Another exception was thrown: There are multiple heroes that share the same tag within a subtree.
this is the error that comes when i run the build.
-
Harshvardhan Joshi over 4 yearscan you post more code epecially where you use
hero
widget, useNavigator
andGesture detector
? -
Jithin Palepu over 4 yearsi updated the code. Its complete now
-
Harshvardhan Joshi over 4 yearsI don't see any gesture detector or Hero widgets used anywhere..
-
Jithin Palepu over 4 yearsthats the problem. I dont know where is the problem, but i think it is in card()
-
Harshvardhan Joshi over 4 yearscan you post all logs from the start so that we can verify the source loacation of the Hero widget.? may be not here if too long.
-
Jithin Palepu over 4 yearssure. Let me update it
-
Jithin Palepu over 4 yearsLet us continue this discussion in chat.
-
Harshvardhan Joshi over 4 yearsI'll appreciate if you take some time to upvote and accept my answer or tell me if facing any problems in the solution.
-