GridView containing Cards are getting Trimmed at the end in Flutter
Solution 1
Just for exploring, you can do height: MediaQuery.of(context).size.height *2,
It shouldn't get cut anymore right?
Solution 1
Your telling your container a given height which is fix for the moment, no matter how many items you got inside your ListView
.
You can for example use ConstrainedBox
with shrinkWrap:true
and manage your max height
ConstrainedBox(
constraints: BoxConstraints(maxHeight: 200, minHeight: 56.0),
child: ListView.builder(
shrinkWrap: true,
...
more info: https://api.flutter.dev/flutter/widgets/ConstrainedBox-class.html
Solution 2
use Slivers
, they are dynamic.
For this you need to customize your structure a little bit. You wrap everything with a CustomScrollView()
, SliverToBoxAdapter()
for fixed elements and SliverList()
for dynamic ones, makes it work like a charm.
Example using CustomScrollView
and SliverToBoxAdapter
:
return SafeArea(
child: CustomScrollView(
//scrollDirection: Axis.vertical,
physics: BouncingScrollPhysics(),
slivers: <Widget>[
// Place sliver widgets here
SliverToBoxAdapter(
child: Padding(
padding: const EdgeInsets.fromLTRB(25, 30, 25, 20),
child: SizedBox(
height: 299,
child: Column(children: <Widget>[
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.center,
children: <Widget>[
...
You can define the height with the SizedBox
height
. Also you can apply nice effects to your whole View on the CustomScrollView
Example Sliverlist:
SliverList(
delegate: SliverChildBuilderDelegate(
(ctx, index) => ChangeNotifierProvider.value(
value: list[index],
child: GestureDetector(
onTap: () {}),
childCount: list.length,
)),
Infos about slivers: https://medium.com/flutterdevs/explore-slivers-in-flutter-d44073bffdf6
Solution 2
The GridView
Widget itself is Scrollable
, So you don't have to wrap it with a Column
and SingleChildScrollView
Widget...
You can now simply scroll down if the Circles goes out of the screen
If you wish all the circles to fit in the screen.. You'll have to use
var mobileHeight = MediaQuery.of(context).size.height
And then the height of each circles will be mobileHeight
divided by the no. of circles vertically.
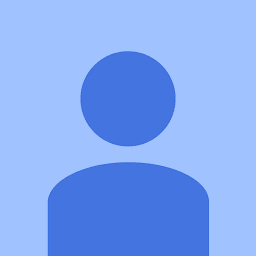
Amit Dubey
Updated on December 29, 2022Comments
-
Amit Dubey over 1 year
I am trying to create one Grid View page with Cards as list elements and the last of the cards are getting cut from the bottom. The following are relevant code snippets:
createListBody.dart
List<String> services = [ 'Demo1', 'Demo2', 'Demo3', 'Demo4', 'Demo5', 'Demo6', 'Demo7', 'Demo8', 'Demo9', 'Demo10', ]; @override Widget build(BuildContext context) { return Container( height: MediaQuery.of(context).size.height, child: GridView.count( crossAxisCount: 2, crossAxisSpacing: 2.0, mainAxisSpacing: 2.0, children: services.map((String serviceName){ return Container( child:Card( color: Colors.grey[500], elevation: 15, semanticContainer: true, shadowColor: palletFuchsia, shape: CircleBorder(), child: InkWell( onTap: (){ print("Tapped "+serviceName); }, child: Center( child: Text( serviceName, style: TextStyle( fontWeight: FontWeight.bold, fontSize: 25 ), ), ), ), ) ); }).toList(), ), ); }
listScreen.dart
Widget build(BuildContext context) { return Scaffold( backgroundColor: palletWhiteDrawer, drawer: Theme( data: Theme.of(context).copyWith( canvasColor: palletYellowDrawer, ), child: CreatDrawerWidget(), ), appBar: CreateAppBar(), body:GestureDetector( behavior: HitTestBehavior.opaque, onTap: () { FocusScope.of(context).requestFocus(new FocusNode()); }, child: SingleChildScrollView( child: Column( mainAxisSize: MainAxisSize.max, crossAxisAlignment: CrossAxisAlignment.center, children: [ ServiceListBody() ], ) ), ), ); }
I am completely new to flutter so, sorry if there is some silly mistake. But any help would be useful. Thank you for your time!
-
Amit Dubey about 3 yearsYes, if using only
GridView
Widget, the page is working just fine, but I want to add oneContainer
with some text above theGridView
, that's why wrapped it in the Column widget -
Amit Dubey about 3 yearsThank you so much, will try these solutions out and will comment the results! :)
-
ibrahimxcool about 3 years@AmitDubey Then you could try wrapping the
GridView
withExpanded
and then you can safely wrap it with aColumn
widget. It it gives an error there are other ways to do it... You can look it up here -
Marcel Dz about 3 yearsYea sure youre welcome :) If it helped you make sure to vote as accepted answer
-
Amit Dubey about 3 yearsmaking
height: MediaQuery.of(context).size.height*2
stops the scrolling completely, and the lower cards are not rendered at all -
Marcel Dz about 3 yearsYea could be because of the rendering errors. By the way I see you used the Gridview. Make sure to use the SliverGrid for perfect solution :-) Check this out: medium.com/flutter/slivers-demystified-6ff68ab0296f You can see in the examples every height is dynamic and scrollable