How to access specific cell in generated List from grid view count in Flutter?
141
Here is a solution, by passing in index of what you want to find and matching that with the index of the cells (as per code below):
import 'package:flutter/material.dart';
class GridExample extends StatefulWidget {
const GridExample({Key? key}) : super(key: key);
@override
_GridExampleState createState() => _GridExampleState();
}
class _GridExampleState extends State<GridExample> {
final a = [133, 118, 117, 116, 115, 114];
@override
Widget build(BuildContext context) {
return Container(
child: GridView.count(
crossAxisCount: 15,
childAspectRatio: 1,
children: List<Widget>.generate(
225,
(index) {
return Stack(
children: [
GridTile(
child: Tile(
index: index,
accessedCell: a[3],
),
),
],
);
},
),
),
);
}
}
class Tile extends StatefulWidget {
Tile({
required this.index,
required this.accessedCell,
Key? key,
}) : super(key: key);
final int index;
final int accessedCell;
@override
State<Tile> createState() => _TileState();
}
class _TileState extends State<Tile> {
bool _isAccessed = false;
@override
Widget build(BuildContext context) {
print(widget.accessedCell);
print(widget.index);
if (widget.accessedCell == widget.index) {
_isAccessed = true;
}
return Container(
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(5),
border: Border.all(color: _isAccessed ? Colors.blue : Colors.red),
),
child:
FittedBox(fit: BoxFit.contain, child: Text(widget.index.toString())),
);
}
}
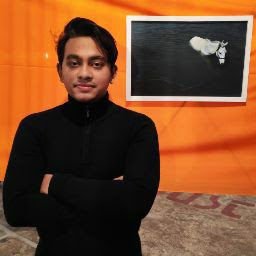
Author by
Gourab Saha
Software Engineering Undergrad | Tech Enthusiast | Amateur FOSS Developer | Learning Data Structures & Algorithms
Updated on December 01, 2022Comments
-
Gourab Saha over 1 year
Here, I have a list. I want to access a specific position on that list in my GridView.count.
Like I have a list, a = [133, 118, 117, 116, 115, 114];
I want to figure it out that in my List.generate where I have generated 225 cells, how can I access a cell which is stored on this list, a = [133, 118, 117, 116, 115, 114]; like I want to access the 4th item(116) of that list from that 225 cells.
a = [133, 118, 117, 116, 115, 114]; child: GridView.count( crossAxisCount: 15, childAspectRatio: 1, children: List<Widget>.generate( 225, (index) { return Stack( children: [ GridTile( child: Container( decoration: BoxDecoration( borderRadius: BorderRadius.circular(5), border: Border.all(color: Colors.black), ), ), ), ], ); }, ), ),
-
Gourab Saha over 2 yearsThank you for answering so fast. It's my mistake, I have to ask the question more specifically. I want to figure it out that in my List<Widget>.generate where I have generated 225 cells, how can I access a cell which is stored on this list, a = [133, 118, 117, 116, 115, 114]; like I want to access the 4th item(116) of that list from that 225 cells.
-
Kdon over 2 yearsOK cool thank you, yes I think I understand now. I have updated my answer: For example to find the value of '113' index ofyour list (eg. a[3]) you can pass that index and match it as per the example. Note if it matches, I changed the border to blue to highlight. You could also pass in a list of values to match multiple cells at once. Hope that helps, thanks.