Boto3: Get EC2 images owned by me
10,143
Solution 1
You should be able to use self for the owner. This is what I use.
boto3conn = boto3.resource("ec2", region_name="us-west-2")
images = boto3conn.images.filter(Owners=['self'])
Solution 2
This will help, It will show you ALL AMI
thats owned
by your aws account
import boto3
client = boto3.client('ec2', region_name='us-east-1')
response = client.describe_images(Owners=['self'])
for ami in response['Images']:
print (ami['ImageId'])
Solution 3
You can use the STS API to get this information.
I found this post talking about it: getting the current user account-id in boto3
So the code you need is:
ec2 = boto3.resource('ec2', region_name='us-east-1')
owner_id = boto3.client('sts').get_caller_identity().get('Account')
filters = [{'Name': 'owner-id', 'Values': [owner_id]}]
images = ec2.images.filter(Filters=filters).all()
Make sure to change the region name to the correct one, or leave it out if you've set it elsewhere in your environment.
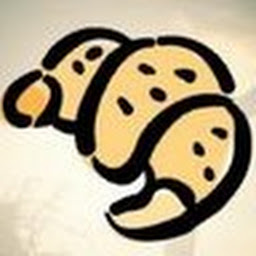
Author by
drootnar
Aspiring full stack developer (Python, servers, aws, databases). Interested in REST Web Services.
Updated on June 07, 2022Comments
-
drootnar almost 2 years
I would like to get all ami images owned by me. I tried something like below:
ec2 = boto3.resource('ec2') owner_id = 'owner_id' filters = [{'Name': 'owner-id', 'Values': [owner_id]}] images = ec2.images.filter(Filters=filters).all()
But I need to put owner_id explicid in the code. Is it any solution to do that automatically from aws credentials?