C# - AsEnumerable Example
Solution 1
After reading the answers, i guess you are still missing a practical example.
I use this to enable me to use linq on a datatable
var mySelect = from table in myDataSet.Tables[0].AsEnumerable()
where table["myColumn"].ToString() == "Some text"
select table;
Solution 2
From the "Remarks" section of the MSDN documentation:
The
AsEnumerable<TSource>
method has no effect other than to change the compile-time type of source from a type that implementsIEnumerable<T>
toIEnumerable<T>
itself.
AsEnumerable<TSource>
can be used to choose between query implementations when a sequence implementsIEnumerable<T>
but also has a different set of public query methods available. For example, given a generic classTable
that implementsIEnumerable<T>
and has its own methods such asWhere
,Select
, andSelectMany
, a call toWhere
would invoke the publicWhere
method ofTable
. ATable
type that represents a database table could have aWhere
method that takes the predicate argument as an expression tree and converts the tree to SQL for remote execution. If remote execution is not desired, for example because the predicate invokes a local method, theAsEnumerable<TSource>
method can be used to hide the custom methods and instead make the standard query operators available.
Solution 3
If you take a look in reflector:
public static IEnumerable<TSource> AsEnumerable<TSource>(this IEnumerable<TSource> source)
{
return source;
}
It basically does nothing more than down casting something that implements IEnumerable.
Solution 4
Nobody has mentioned this for some reason, but observe that something.AsEnumerable()
is equivalent to (IEnumerable<TSomething>) something
. The difference is that the cast requires the type of the elements to be specified explicitly, which is, of course, inconvenient. For me, that's the main reason to use AsEnumerable()
instead of the cast.
Solution 5
AsEnumerable() converts an array (or list, or collection) into an IEnumerable<T> of the collection.
See http://msdn.microsoft.com/en-us/library/bb335435.aspx for more information.
From the above article:
The AsEnumerable<TSource>(IEnumerable<TSource>) method has no effect other than to change the compile-time type of source from a type that implements IEnumerable<T> to IEnumerable<T> itself.
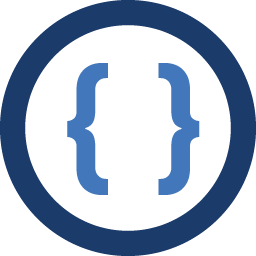
Admin
Updated on August 20, 2022Comments
-
Admin over 1 year
What is the exact use of AsEnumerable? Will it change non-enumerable collection to enumerable collection?.Please give me a simple example.