Need to make an enumeration of type double in c#
Solution 1
You can't make it an enumeration.
http://msdn.microsoft.com/en-us/library/y94acxy2.aspx
One of possibilities:
public static class MyPseudoEnum
{
public static readonly double FirstVal = 0.3;
public static readonly double SecondVal = 0.5;
}
Solution 2
I guess it depends on how much trouble you want to go to. You can convert a double to a long, so if you converted your double values to long integers in a separate program, and output the constant values, you could then use them in your program.
I would strongly discourage this, by the way, but sometimes you gotta do what ya gotta do.
First, write a program to convert your doubles to longs:
Console.WriteLine("private const long x1 = 0x{0:X16}L;",
BitConverter.DoubleToInt64Bits(0.5));
Console.WriteLine("private const long x2 = 0x{0:X16}L;",
BitConverter.DoubleToInt64Bits(3.14));
Now, add that output to your program and create the enum:
private const long x1 = 0x3FE0000000000000L;
private const long x2 = 0x40091EB851EB851FL;
enum MyEnum : long
{
val1 = x1,
val2 = x2
}
When you want to test a double value against the enum, you'll have to call BitConverter.DoubleToInt64Bits()
, and cast the result to your enum type. To convert the other way, call BitConverter.Int64BitsToDouble()
.
Solution 3
No, enums are essentially integer values.
I don't know about your particular problem you're solving but you could do a generic dictionary.
Of course, comparison of doubles is always fun, particularly when they differ way down in the decimals places. Might be interesting if you try to compare the results of a calculation (for equality) against a known double. Just a caution, if that is what you might need to do.
Solution 4
Enum types have to be integral types, with the exception of char
. This doesn't include double
, so you'll need to make a lookup table or a set of constants.
Solution 5
I think byte, sbyte, short, ushort, int, uint, long, or ulong are your only options.
Why, as a matter of interest? Maybe we can work round it.
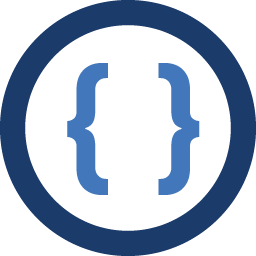
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
How can I create an enum of type double?
Is it possible or do I have to create some kind of collection and hash?