C# Convert object of type array to T, where T is an array?
11,377
Solution 1
This worked for me:
public T[] GetValue<T>(object o)
{
Converter<int, T> c = new Converter<int, T>(input => (T)System.Convert.ChangeType(input, typeof(T)));
return (T[])Array.ConvertAll<int, T>((int[])o, c);
}
I hope this helps!
Solution 2
Try this:
public T[] getValue<T>(object o)
{
return (T[])Convert.ChangeType(o, typeof(T[]));
}
Use like this:
object doubleArr = new Double[] {1.3, 1.5, 1.7};
var returnedValue = getValue<double>(doubleArr);
Note that if you pass in the wrong type for the template, it will fail at run time
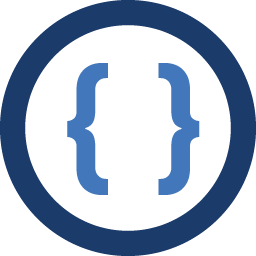
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
It'd be really good if I could get an object to array conversion working.
Steps:
- We get passed an array from an external source. The array is boxed in an object. Typically the object is an int[] or a double[], but we normally want double[].
- Convert it to an array of Type T.
- return the converted type.
For starters, this works fine
double[] r=Array.ConvertAll<int,double>((int[])o,Convert.ToDouble)
but this doesn't (assume that T is "double") e.g. double[] R=getValue(o);
public T[] getValue<T>(object o){ // ... some logic... return (T[])Array.ConvertAll<int,double>((int[])o,Convert.ToDouble)
Is there a where constraint that can be used? Array is a "Special Object", so we can't use that as a constraint.
Is this possible in .net without resorting to IL? Is it possilbe if we do resort to IL?
thanks, -Steven