Java Instantiate new Map.Entry-array
10,236
Do you need to have an array? You can do the following with a List
:
public static void main(String[] args) {
array = new ArrayList<Map.Entry<Integer, Integer>>();
}
private static List<Map.Entry<Integer, Integer>> array;
Alternately, you can instantiate the non generic type, and cast to the generic type:
public static void main(String[] args) {
array = (Map.Entry<Integer, Integer>[])new Map.Entry[1];
}
private static Map.Entry<Integer, Integer>[] array;
However, this will give you warnings, and is generally not preferred.
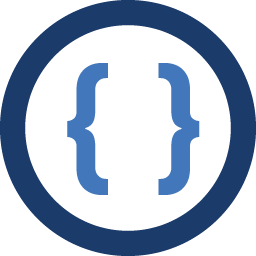
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm having problems casting an object array to a key-value pair array, with generic types for the key and value objects. Here is a minimal example.
public class Main { public static void main(String[] args) { array = (Map.Entry<Integer, Integer>[]) new Object[1]; } private static Map.Entry<Integer, Integer>[] array; }
Changing Map.Entry to a class (rather than interface) doesn't do the trick either.
Error trace:
run: Exception in thread "main" java.lang.ClassCastException: [Ljava.lang.Object; cannot be cast to [Ljava.util.Map$Entry; at lab2.Main.main(Main.java:13) Java Result: 1