C# Entity Framework: Keyword not supported: 'port'
Solution 1
The argument of the used base DbContext
constructor is called nameOrConnectionString
. Hence it supports a name of a connection string from the configuration file, or like in your case an actual connection string.
The problem with the later is that it doesn't allow specifying the provider name as with the former coming from the configuration, in which case EF uses the one specified in the defaultConnectionFactory
configuration element, which in your case is System.Data.Entity.Infrastructure.SqlConnectionFactory
, in other words - Sql Server, hence the port
not supported exception.
There are several ways to fix the issue.
(A) Change the defaultConnectionFactory
configuration:
<defaultConnectionFactory type="MySql.Data.Entity.MySqlConnectionFactory, MySql.Data.Entity.EF6"></defaultConnectionFactory>
(B) Use named configuration connection string and specify explicitly the provider:
<connectionStrings>
<add name="MyDB" providerName="MySql.Data.MySqlClient" connectionString="server=myservername;port=3306;uid=myaccount;database=mydb;pwd=mypwd123" />
</connectionStrings>
and change the constructor to
public MyDB()
{
// ...
}
or if the name is different than your DbContext
derived class name:
public MyDB() : base(connection_string_name)
{
// ...
}
(C) Use DbConfigurationTypeAttribute
:
[DbConfigurationType(typeof(MySql.Data.Entity.MySqlEFConfiguration))]
public class MyDB : DbContext
{
// ...
}
Solution 2
I had this problem whilst developing a Web Application on Core 2. I had to change the default database connection used from SqlServer to MySql in the Startup.cs file where the application is configured.
Solution 3
The error similar to the one listed above comes while working with ASP.net core and Database. The default database Provider with the ASP.net core is the SQL Server but, in case you are using a different provider for example, PostgreSQL and didn't correctly write or configure the DBContext code in the startup.cs
For example - Following code is written with an intent to connect to PostgresSQL then it will result in error ArgumentException: Keyword not supported: 'port'.
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
var connection = @"Server=localhost;Port=5432;Database=NewDB;User Id=xxxxx;Password=cr@aaaa;";
services.AddDbContext<BloggingContext>(options => options.UseSqlServer(connection));
// ...
}
And the reasons is user is trying to connect to PostgreSQL but did change the default Option UseSQLServer after configuring the PostgreSQL string.
To fix the issue change the option
options.UseSqlServer(connection)) -> options.UseNpgsql(connection))
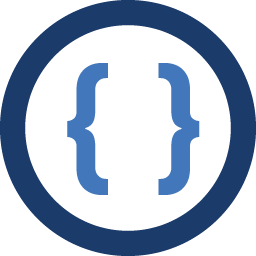
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
Hello I have more than one project connecting to a certain DB that is CodeFirst Entity Framework.
All Projects are able to connect successfully except for one stubborn one.
The error I am getting is:
Keyword not supported: 'port'
I have looked through countless stackoverflow questions, mysql forums, entity framework forums etc. including:
MappingException Edm.String not compatible with SqlServer.varbinary
Keyword not supported in MySQL's connection string
Keyword not supported: 'metadata' + MySQL
My connection string looks like:
server=myservername;port=3306;uid=myaccount;database=mydb;pwd=mypwd123
My db.cs file looks like:
public partial class MyDB : DbContext { public MyDB () : base("server=myservername;port=3306;uid=myaccount;database=mydb;pwd=mypwd123") { Logger.Trace("test123"); } public virtual DbSet<MyItem> MyItems { get; set; } protected override void OnModelCreating(DbModelBuilder modelBuilder) { modelBuilder.Entity<MyItem>() .Property(e => e.Content) .IsUnicode(false); } }
When I remove the
port:3306
from the connection string I get this:System.Data.Entity.Core.MappingException: Schema specified is not valid. Errors: (8,12) : error 2019: Member Mapping specified is not valid. The type 'Edm.DateTime[Nullable=False,DefaultValue=,Precision=]' of member 'Time' in type 'something.Model.MyItem' is not compatible with 'SqlServer.timestamp[Nullable=False,DefaultValue=,MaxLength=8,FixedLength=True,StoreGeneratedPattern=Identity]' of member 'time' in type 'CodeFirstDatabaseSchema.MyItem'. at System.Data.Entity.Core.Mapping.StorageMappingItemCollection.Init(EdmItemCollection edmCollection, StoreItemCollection storeCollection, IEnumerable`1 xmlReaders, IList`1 filePaths, Boolean throwOnError) at System.Data.Entity.Core.Mapping.StorageMappingItemCollection..ctor(EdmItemCollection edmCollection, StoreItemCollection storeCollection, IEnumerable`1 xmlReaders) at System.Data.Entity.ModelConfiguration.Edm.DbDatabaseMappingExtensions.ToStorageMappingItemCollection(DbDatabaseMapping databaseMapping, EdmItemCollection itemCollection, StoreItemCollection storeItemCollection) at System.Data.Entity.ModelConfiguration.Edm.DbDatabaseMappingExtensions.ToMetadataWorkspace(DbDatabaseMapping databaseMapping) at System.Data.Entity.Internal.CodeFirstCachedMetadataWorkspace..ctor(DbDatabaseMapping databaseMapping) at System.Data.Entity.Infrastructure.DbCompiledModel..ctor(DbModel model) at System.Data.Entity.Internal.LazyInternalContext.CreateModel(LazyInternalContext internalContext) at System.Data.Entity.Internal.RetryLazy`2.GetValue(TInput input) at System.Data.Entity.Internal.LazyInternalContext.InitializeContext() at System.Data.Entity.Internal.InternalContext.GetEntitySetAndBaseTypeForType(Type entityType) at System.Data.Entity.Internal.Linq.InternalSet`1.Initialize() at System.Data.Entity.Internal.Linq.InternalSet`1.get_InternalContext() at System.Data.Entity.Internal.Linq.InternalSet`1.ActOnSet(Action action, EntityState newState, Object entity, String methodName) at System.Data.Entity.Internal.Linq.InternalSet`1.Add(Object entity) at System.Data.Entity.DbSet`1.Add(TEntity entity) at MyFunction(Int32 userId, String id, String type, String contentJsonString) in
I am using MySql Connector and not Sql Server...
I am completely stumped by this as well as the rest of my team.
Edit: Here is my Web.Config
<?xml version="1.0"?> <configuration> <configSections> <section name="entityFramework" type="System.Data.Entity.Internal.ConfigFile.EntityFrameworkSection, EntityFramework, Version=6.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" requirePermission="false"/> </configSections> <appSettings file="config-sources\app-settings.config"/> <system.web> <compilation debug="true" targetFramework="4.5.2"> <assemblies> <add assembly="System.Data.Entity, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" /> </assemblies> </compilation> <httpRuntime targetFramework="4.5.1"/> </system.web> <connectionStrings configSource="config-sources\ef-connection-strings.config"/> <runtime> <assemblyBinding xmlns="urn:schemas-microsoft-com:asm.v1"> <dependentAssembly> <assemblyIdentity name="MySql.Data" publicKeyToken="C5687FC88969C44D" culture="neutral" /> <bindingRedirect oldVersion="0.0.0.0-6.8.3.0" newVersion="6.8.3.0" /> </dependentAssembly> <dependentAssembly> <assemblyIdentity name="Newtonsoft.Json" publicKeyToken="30ad4fe6b2a6aeed" culture="neutral"/> <bindingRedirect oldVersion="0.0.0.0-8.0.0.0" newVersion="8.0.0.0"/> </dependentAssembly> <dependentAssembly> <assemblyIdentity name="Autofac" publicKeyToken="17863af14b0044da" culture="neutral"/> <bindingRedirect oldVersion="0.0.0.0-3.3.0.0" newVersion="3.3.0.0"/> </dependentAssembly> <dependentAssembly> <assemblyIdentity name="EntityFramework" publicKeyToken="b77a5c561934e089" culture="neutral"/> <bindingRedirect oldVersion="0.0.0.0-6.0.0.0" newVersion="6.0.0.0"/> </dependentAssembly> <dependentAssembly> <assemblyIdentity name="System.Net.Http.Primitives" publicKeyToken="b03f5f7f11d50a3a" culture="neutral"/> <bindingRedirect oldVersion="0.0.0.0-4.2.29.0" newVersion="4.2.29.0"/> </dependentAssembly> </assemblyBinding> </runtime> <entityFramework> <defaultConnectionFactory type="System.Data.Entity.Infrastructure.SqlConnectionFactory, EntityFramework" /> <providers> <provider invariantName="MySql.Data.MySqlClient" type="MySql.Data.MySqlClient.MySqlProviderServices, MySql.Data.Entity.EF6" /> <provider invariantName="System.Data.SqlClient" type="System.Data.Entity.SqlServer.SqlProviderServices, EntityFramework.SqlServer" /> </providers> </entityFramework> <system.webServer> <handlers> <remove name="ExtensionlessUrlHandler-Integrated-4.0"/> <add name="ExtensionlessUrlHandler-Integrated-4.0" path="*." verb="POST,HEAD,GET" type="System.Web.Handlers.TransferRequestHandler" resourceType="Unspecified" requireAccess="Script" preCondition="integratedMode,runtimeVersionv4.0" /> </handlers> <security> <requestFiltering> <verbs> <add verb="POST" allowed="true"/> </verbs> </requestFiltering> </security> <defaultDocument> <files> <add value="webhook.ashx"/> </files> </defaultDocument> </system.webServer> </configuration>
-
Admin almost 7 yearsThanks a lot! I ended up using option (A) although I wanted to use option (C) I couldn't get an import of
MySql.Data.Entity
from anywhere... Might have to update my MySqlConnector version for this -
Haikal Nashuha almost 6 yearsWhere should I put (A) solution? In csproj?
-
Ivan Stoev almost 6 years@HaikalNashuha (A) is for App.config (or Web.config) file