c++ equivalent to python append method for lists
13,962
Solution 1
You need a vector, do something like this:
#include <vector>
void funct() {
std::vector<int> myList;
for(int i = 0; i < 10; i++)
myList.push_back(10);
}
See http://cplusplus.com/reference/stl/vector/ for more information.
Solution 2
For list Use std::list::push_back
If you are looking for a array equivalent of C++, You should use std::vector
vector also has a std::vector::push_back method
Solution 3
You should use vector:
vector<int> v;
for(int i = 0; i < 10; i++)
v.push_back(i);
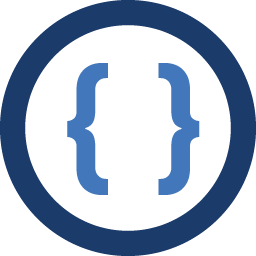
Author by
Admin
Updated on July 03, 2022Comments
-
Admin almost 2 years
I'm learning c++ coming from a background in python.
I'm wondering is there a way to append items to a list in c++?
myList = [] for i in range(10): myList.append(i)
Is there something like this in c++ that you can do to an array?