C#: How do I iterate the contents in a DataColumn?
Solution 1
You iterate the rows, not the column
DataColumn SETTINGS = dt.Columns["DEFAULTSETTINGS"];
foreach(DataRow row in dt.Select())
{
object columnValue = row[SETTINGS];
// Do something with columnValue
}
Solution 2
There's no need to use Linq or iterate manually through your DataRows as plain old ADO.NET has a couple of solutions such as DataTable.Select and DataView.RowFilter.
string value = "default";
bool found = dt.Select("DEFAULTSETTINGS = " + value).Length > 0;
It's obvious that once you get the filtered rows, you can do whatever you want with them. In my example, I just checked to see if any row was within the criteria but you can manipulate them how you see fit.
Solution 3
You can use linq in .net 3.5:
DataColumn col = dt.Columns["DEFAULTSETTINGS"];
object value = //expected value
bool valueExists = dt.AsEnumerable().Any(row => row.Field<object>(col).Equals(value));
EDIT: It seems from the comments you're trying to see if the 'DEFAULTSETTINGS' column contains the string 'default'. The Field extension method simply casts the object in the datarow at that column into the given type, so you can change the type parameter rather than use ToString(). So for your example you can probably use this:
DataColumn col = dt.Columns["DEFAULTSETTINGS"];
bool valueExists = dt.AsEnumerable().Any(row => "default".Equals(row.Field<string>(col), StringComparison.OrdinalIgnoreCase);
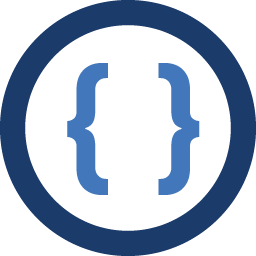
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I have a database column I simply need to check to see if a value is there.
DataTable dt = masterDataSet.Tables["Settings"]; DataColumn SETTINGS = dt.Columns["DEFAULTSETTINGS"];
I just need to iterate over the values in this column to see if a value exists. Help