c++ - <unresolved overloaded function type>
65,272
The problem here is that f2
is a method on Mat
, while f
is just a free function. You can't call f2
by itself, it needs an instance of Mat
to call it on. The easiest way around this might be:
printf("%d\n", test([=](int v){return this->f2(v);}, 5));
The =
there will capture this
, which is what you need to call f2
.
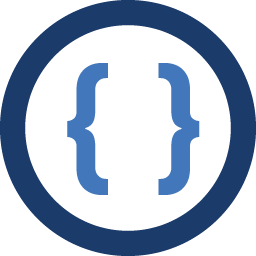
Author by
Admin
Updated on September 17, 2020Comments
-
Admin over 3 years
In my class called
Mat
, I want to have a function which takes another function as a parameter. Right now I have the 4 functions below, but I get an error in when calling print(). The second line gives me an error, but I don't understand why, since the first one works. The only difference is functionf
is not a member of the classMat
, butf2
is. The failure is:error: no matching function for call to Mat::test( < unresolved overloaded function type>, int)'
template <typename F> int Mat::test(F f, int v){ return f(v); } int Mat::f2(int x){ return x*x; } int f(int x){ return x*x; } void Mat::print(){ printf("%d\n",test(f ,5)); // works printf("%d\n",test(f2 ,5)); // does not work }
Why does this happen?
-
2to1mux about 11 yearsSince f2 and print are both member functions of Mat, isn't print allowed to call f2 without referencing a Mat object?
-
Luchian Grigore about 11 yearsWow, this works (with a few tweaks from the original code). +1 from me
-
Johannes Schaub - litb about 11 years-1 . the error message says that the error happens because of an overloaded function, and not because the function template's function call syntax is wrong (which is what you say).
-
2to1mux about 11 years@JohannesSchaub-litb That isn't what he is saying. He's saying that f2 can only be called via an instance of a Mat object. This solution solves the issue (Although, I actually think it solves the original compiler error for a different reason than the one stated by Barry).
-
Barry about 11 yearsI think in this case the compiler error is not the best.
f2
just isn't a function,&Mat::f2
is. If you tried to pass in that instead, you'd get a compile error about invalid call syntax. -
Admin about 11 yearsThis also gives me an error: "no matching function for call to 'Mat::test(Mat::print()::< lambda(int)>, int)'"
-
fritzelr over 3 yearsMinor nit: according to cppreference.com:
The current object (*this) can be implicitly captured if either capture default is present. If implicitly captured, it is always captured by reference, even if the capture default is =. [[The implicit capture of *this when the capture default is = is deprecated. (since C++20)]]
. So the capture should use[&]
. -
Jose Areas about 3 yearsthe question is about using functions as another function input. The issue you had is because you were passing a function instead of its return as a param to myFunction - value.x - "x" is a property of object "value". Which in your case, it is a method - value.x() - this is a call to the method "x"