C++ Passing Struct Address
Solution 1
Yes, your code is compilable other than the missing semicolons in the defintion of struct stuff
. I'm not quite sure exactly what you're asking about passing the function and the actual function call, but I think you're wondering why the function call uses &fnc
, but the parameter is stuff *pm
? In that case, the fnc
variable declared is a plain stuff
. It is not a pointer, it refers to the actual instance of that struct.
Now the multiply
function is declared as taking a stuff*
-- a pointer to a stuff
. This means that you can't pass fnc
directly -- it's a stuff
and multiply
expects a *stuff
. However, you can pass fnc
as a stuff*
by using the & operator to take the address, and &fnc
is a valid stuff*
that can be passed to multiply
.
Once you're in the multiply
function, you now have a stuff*
called pm
. To get the one
and two
variables from this stuff*
, you use the pointer to member operator (->
) since they are pointers to a stuff
and not a plain stuff
. After obtaining those values (pm->one
and pm->two
), the code then multiples them together before printing them out (pm->one * pm->two
).
Solution 2
The *
and &
operands mean different things depending on whether they describe the type or describe the variable:
int x; // x is an integer
int* y = &x; // y is a pointer that stores the address of x
int& z = x; // z is a reference to x
int a = *y; // a in an integer whose value is the deference of y
Your pm
variable is declared as a pointer, so the stuff
type is modified with *
. Your fnc
variable is being used (namely for its address), and thus the variable itself is marked with &
.
You can imagine the above examples as the following (C++ doesn't actually have these, so don't go looking for them):
int x;
pointer<int> y = addressof(x);
reference<int> z = x;
int a = dereference(y);
It the difference between describing a type and performing an operation.
Solution 3
In
void multiply(const stuff * pm){
cout << pm->one * pm->two;
}
The stuff * pm
says that pm is an address of a stuff struct.
The
&fnc
says "the address of fnc
".
When a variable is declared like:
stuff *pm;
it tells us that pm
should be treated like an address whose underlying type is stuff
.
And if we want to get the address of a variable stuff fnc
, we must use
&fnc
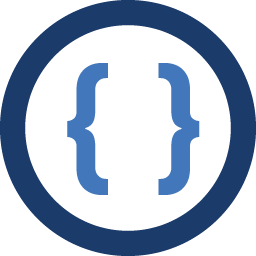
Admin
Updated on June 27, 2022Comments
-
Admin almost 2 years
Im a little bit confused about passing structs into functions. I understand pointers and everything.
But for instance:
struct stuff { int one int two }; int main{ stuff fnc; fnc.two = 2; fnc.one = 1; multiply(&fnc); } void multiply(const stuff * pm){ cout << pm->one * pm->two; }
First of all....am i even doing this right. And second of all, why do we use the address operator when we pass the function, but use the * pointer operator in the actual function call? Im confused?
-
Admin over 13 yearsYa thanks, it was just an example I threw together. I was just confused at the usage of * and & operators
-
Admin over 13 yearsYes this is precisely what I was asking. Thanks, my wording was terribly messed up in my question lol
-
San Jacinto over 13 yearsI think this is the only thing that actually answers the question.