Call javascript from dart native/flutter
Solution 1
Embed a WebView in your app and use it to run JavaScript:
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:webview_flutter/webview_flutter.dart';
main() {
runApp(_Test());
}
class _Test extends StatelessWidget {
final completer = Completer<WebViewController>();
@override
Widget build(BuildContext context) {
var js = '1+1';
return MaterialApp(
home: Scaffold(
body: Stack(
children: [
WebView(
javascriptMode: JavascriptMode.unrestricted,
onWebViewCreated: (controller) {
if (!completer.isCompleted) completer.complete(controller);
},
),
Center(
child: FutureBuilder(
future: completer.future.then((controller) {
return controller.runJavascriptReturningResult(js);
}),
builder: (context, snapshot) {
if (snapshot.hasError) throw snapshot.error!;
if (!snapshot.hasData) return Container();
return Text('$js=${snapshot.data}', style: Theme.of(context).textTheme.headline2);
},
),
),
],
),
),
);
}
}
Solution 2
If all you need is the Circom library, someone has already implemented it in Dart. You can check out the Hermez SDK package and see how they did it. Specifically, in this file.
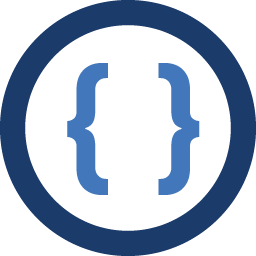
Admin
Updated on January 04, 2023Comments
-
Admin over 1 year
I've seen that calling js is definitely possible from dart web using the dart js package, however haven't found a way to do this from dart native. For some more context - I have an npm package written in javascript and I would like to invoke this from a flutter mobile app. Calling the javascript directly or somehow wrapping it in dart or some other way are all fine for me, however rewriting it in dart is not possible as said npm package depends on a very specific cryptography javascript package to which no equivalent exists in dart, and recreating said cryptography package would bear a huge workload and significant security risks. Thank you in advance!
-
Richard Heap almost 2 yearsIt's not possible. You can either port the library to Dart (which cryptography function is missing?) or find the same in C and call it via FFI.
-
Richard Heap almost 2 yearsWhat's the "very specific crypto package"?
-
Elliot Solskjaer almost 2 yearssnarkJS, circomlib, and the circom compiler
-
Richard Heap almost 2 yearsIt looks like FFI to the C++ equivalents might be possible.
-
-
Elliot Solskjaer almost 2 yearsGood to know in case we do decide on a future rewrite - upvoted!
-
triple7 almost 2 yearsPersonally, I would avoid spinning up a web view just so I can run some arbitrary code in it. It's just opening myself to potential memory and security leaks, and it just "feels wrong". You know?
-
Elliot Solskjaer almost 2 yearsAbsolutely agree, I definitely also view this more as a short-term fix now that I know that a rewrite is possible thanks to this package - thanks again!