Camera Preview is too dark in low light android
Solution 1
To solve the problem you have to unlock autoExposureCompensation and set to the MAX value:
Camera.Parameters params = mCamera.getParameters();
params.setExposureCompensation(params.getMaxExposureCompensation());
if(params.isAutoExposureLockSupported()) {
params.setAutoExposureLock(false);
}
mCamera.setParameters(params);
According to Android Develop > Reference > Camera.Parameters
Changing the white balance mode with setWhiteBalance(String) will release the auto-white balance lock if it is set.
Exposure compensation, AE lock, and AWB lock can be used to capture an exposure-bracketed burst of images, for example. Auto-white balance state, including the lock state, will not be maintained after camera release() is called.
Locking auto-white balance after open() but before the first call to startPreview() will not allow the auto-white balance routine to run at all, and may result in severely incorrect color in captured images.
So be careful and use the mentioned code after the first call to startPreview()
Solution 2
Try the below code. It worked for me.
previewRequestBuilder.set(CaptureRequest.CONTROL_AE_TARGET_FPS_RANGE, getRange());//This line of code is used for adjusting the fps range and fixing the dark preview
previewRequestBuilder.set(CaptureRequest.CONTROL_AE_LOCK, false);
previewRequestBuilder.set(CaptureRequest.CONTROL_AF_MODE, CaptureRequest.CONTROL_AE_PRECAPTURE_TRIGGER_START);
getRange() function as belw:
private Range<Integer> getRange() {
CameraManager mCameraManager = (CameraManager) getSystemService(Context.CAMERA_SERVICE);
CameraCharacteristics chars = null;
try {
chars = mCameraManager.getCameraCharacteristics(cameraId);
} catch (CameraAccessException e) {
e.printStackTrace();
}
Range<Integer>[] ranges = chars.get(CameraCharacteristics.CONTROL_AE_AVAILABLE_TARGET_FPS_RANGES);
Range<Integer> result = null;
for (Range<Integer> range : ranges) {
int upper = range.getUpper();
// 10 - min range upper for my needs
if (upper >= 10) {
if (result == null || upper < result.getUpper().intValue()) {
result = range;
}
}
}
return result;
}
Solution 3
What worked for me, after hours of testing with different settings was to reduce Frames per Second.
By doing so I guess the Automatic Exposure correction has enough time to compute the best settings for the available light.
So, reducing from 30 fps to 10 fps made it.
mCameraSource = new CameraSource.Builder(context, detector)
.setRequestedPreviewSize(640, 480)
.setFacing(CameraSource.CAMERA_FACING_FRONT)
.setRequestedFps(10.0f)
.build();
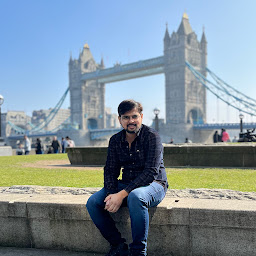
Likith Ts
Updated on July 26, 2022Comments
-
Likith Ts almost 2 years
My camera app preview is too dark in low light. If I open my google camera it will increase brightness inside the preview so that our face is visible to take photos. But my preview is completely dark. I have handled the brightness and lightsensor. My Lightsensor works when is some light. I need to make preview is visible. Let me what should I have to handle?
public void initialBrightness() { try { brightnessMode = Settings.System.getInt(getContentResolver(), Settings.System.SCREEN_BRIGHTNESS_MODE); } catch (Settings.SettingNotFoundException e) { e.printStackTrace(); } if (brightnessMode == Settings.System.SCREEN_BRIGHTNESS_MODE_AUTOMATIC) { Settings.System.putInt(getContentResolver(), Settings.System.SCREEN_BRIGHTNESS_MODE, Settings.System.SCREEN_BRIGHTNESS_MODE_MANUAL); brightnessModeAuto = true; } Settings.System.putInt(this.getContentResolver(), Settings.System.SCREEN_BRIGHTNESS, 95); WindowManager.LayoutParams lp = getWindow().getAttributes(); lp.screenBrightness = 100; getWindow().setAttributes(lp); }
I'm calling this method in onCreate method before camera preview class is called.
-
Likith Ts about 9 yearsI have already implemented SCENE_MODE_NIGHT. And I have implemented when starting the preview. If I'm wrong correct me with some example code.
-
King of Masses about 9 yearsI solved it by giving variable fps to the Camera. First call Camera.getParameter.getSupportedPreviewFpsRange(); In my case I got (15000 to 15000),(24000 to 24000),(30000 to 30000) and (15000,30000). I tried setting range from 15000 to 30000. Make sure that you are passing to different number.
-
Shailendra Madda over 6 yearsHow can we do it if it is in camera2?
-
Innocent over 5 yearswhat is .serRequestedFps here ?
-
Innocent over 5 yearsHow can I set frames per miliseconds ?
-
Clement Osei Tano about 5 yearsThis is the only solution that has worked for me so far. But the preview I'm getting looks like night mode
-
Rémi P over 4 yearsIf you found an answer @ShylendraMadda I am interested in ! I have the exact same problem on CameraX new library