Can't convert expression to float
Solution 1
This is a classic example of what is said in PEP-8 about wildcard imports:
Wildcard imports (
from <module> import *
) should be avoided, as they make it unclear which names are present in the namespace, confusing both readers and many automated tools.
The problem is that you need to work with sympy.log
class, but using math.log
function instead which works on float
objects, not Symbol
objects.
When you write
from sympy import *
you are importing in your module namespace everything that sympy
package providing at the top level (and there are a lot of stuff, much of that you don't need at all), including sympy.log
class.
After next statement
from math import *
you are importing everything in math
module, including math.log
, which overwrites previously imported sympy.log
class.
Considering this your example may be written like
import sympy
def h(x):
return sympy.log(0.485022 * x)
x = sympy.symbols('x')
h_x = h(x)
hprime = h_x.diff(x)
print(hprime)
gives us
1.0/x
P. S.: I've removed math
import since it is not used in given example.
Solution 2
The problem here is that both the sympy
and the math
package define a function called log
.
Importing them as from sympy import *
and then from math import *
overrides the sympy.log
with math.log
.
Better always use import sympy
and then call your functions sympy.log
or (if as lazy as me) do import sympy as sym
and then sym.log
. Be sure to do so with the math package as well. This method will save you a lot of hassle in the future and makes your code easier to understand for others.
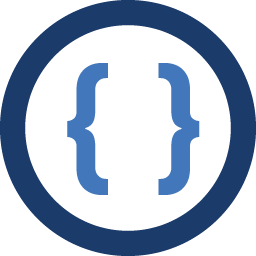
Admin
Updated on June 28, 2022Comments
-
Admin almost 2 years
I'm trying to learn the ins and outs of symbolic manipulation in python (I'm a beginner).
I have the following basic code, and the output is giving me an error telling me that it "can't convert expression to float".
What's wrong with this code:
from sympy import * from math import * def h(x): return log(0.75392 * x) x = symbols('x') hprime = h(x).diff(x) print(hprime)