How do you evaluate a derivative in python?
Your function fprime
is not the derivative. It is a function that returns the derivative (as a Sympy expression). To evaluate it, you can use .subs
to plug values into this expression:
>>> fprime(x, y).evalf(subs={x: 1, y: 1})
3.00000000000000
If you want fprime
to actually be the derivative, you should assign the derivative expression directly to fprime
, rather than wrapping it in a function. Then you can evalf
it directly:
>>> fprime = sym.diff(f(x,y),x)
>>> fprime.evalf(subs={x: 1, y: 1})
3.00000000000000
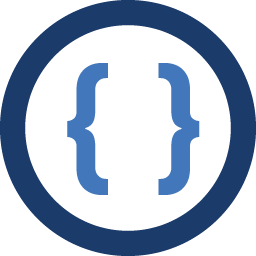
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
I'm a beginner in python. I've recently learned about Sympy and its symbolic manipulation capabilities, in particular, differentiation. I am trying to do the following in the easiest way possible:
- Define f(x,y) = x^2 + xy^2.
- Differentiate f with respect to x. So f'(x,y) = 2x + xy^2.
- Evaluate the derivative, e.g., f'(1,1) = 2 + 1 = 3.
I know how to do 1 and 2. The problem is, when I try to evaluate the derivative in step 3, I get an error that python can't calculate the derivative. Here is a minimal working example:
import sympy as sym import math def f(x,y): return x**2 + x*y**2 x, y = sym.symbols('x y') def fprime(x,y): return sym.diff(f(x,y),x) print(fprime(x,y)) #This works. print(fprime(1,1))
I expect the last line to print 3. It doesn't print anything, and says "can't calculate the 1st derivative wrt 1".
-
Quartal almost 7 years@user46944 I haven't used sympy so I'm not sure how it works exactly but if you changed it to something like this def fprime(xValue, y): return sym.diff(f(valueX, y),x) I think it should work
-
BrenBarn almost 7 years@user46944: No, because
fprime
is still a sympy expression, and you don't evaluate sympy expressions by calling them. You have to useevalf
. -
Quartal almost 7 years@user46944 x, y = sym.symbols('x y') this line sets x value right?
-
Quartal almost 7 years@user46944 In the function def f(x,y): return x2 + x*y2 x takes the value which you call the function with
-
BrenBarn almost 7 years@user46944: For that you could do
fprime.subs(y, 1)
. I don't think there is a way to make a sympy object that can be manipulated symbolically (e.g., differentiated) but can also be called to substitute values like that. To substitute values you need to to use.subs
or.evalf
. -
BrenBarn almost 7 years@user46944: Sympy expressions and Python functions are two different things. Sympy expressions can be differentiated, but not called. Python functions can be called, but not differentiated. Your original question doesn't say anything about plotting or these other issues you're raising in the comments. If you have some additional question, you should probably ask it as a separate question, including in the text of that question the code you're trying to use.
-
BrenBarn almost 7 years@user46944: It's not differentiating your function, it's differentiating the return value of your function, which is a sympy expression.