Can't SET/GET with NodeJS and Redis
12,177
Solution 1
First, you need to know if your redis client is connected. You can check with console.log(client) and you will see a varaible called "connected". You should see "true"
If you want to use redis in node you should use something like this
client.set("test","val", function(err) {
if (err) {
// Something went wrong
console.error("error");
} else {
client.get("test", function(err, value) {
if (err) {
console.error("error");
} else {
console.log("Worked: " + value);
}
});
}
});
Keep in mind that all redis function are asynchronous.
Solution 2
If it's not obvious from the accepted answer, the reason the posted code is failing is due to the asynchronicity.
You're calling get() before set() has completed.
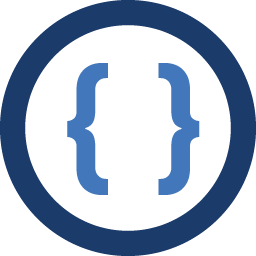
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I want to write a ueberDB Redis-Handler for my Etherpad.
I absolutely do not understand my problem because with PHP I can set and get key/values without any problems. It only does not work with NodeJS.
Here is a example code:
var redis = require("redis"); client = redis.createClient(); client.on("error", function (err) { console.log("Error " + err); }); client.set("test", "string val", redis.print); console.log(client.get("test"));
What do I wrong? Maybe somebody has a tip for me.
-
malletjo over 12 yearsWell, be sure you're connected to redis and you can check the content of redis with the command "redis-cli" from CLI
-
Admin over 12 yearsredis 127.0.0.1:6379> set test test OK redis 127.0.0.1:6379> get test "test" redis 127.0.0.1:6379> exit [root@123 tmp]# node 1.js [root@123 tmp]#
-
malletjo over 12 yearsFeel free to accept this answer. Since you're new: read the faq (stackoverflow.com/faq)
-
truthadjustr almost 8 yearshi Sir, what are the js or nodejs way of working around this asynchronicity that you mentioned. Seems, like the JS's event-driven way of doing things is getting in the way here...