Can I recover a nano process from a previous terminal?
Solution 1
Reading nano man page, and some search, I found:
In some cases nano will try to dump the buffer into an emergency file. This will happen mainly if nano receives a SIGHUP or SIGTERM or runs out of memory. It will write the buffer into a file named nano.save if the buffer didn't have a name already, or will add a ".save" suffix to the current filename. If an emergency file with that name already exists in the current directory, it will add ".save" plus a number (e.g. ".save.1") to the current filename in order to make it unique. In multibuffer mode, nano will write all the open buffers to their respective emergency files.
So you should maybe already have such a file waiting for you, somewhere on your system.
find /likely/path -mtime -1 -print | egrep -i '\.save$|\.save\.[1-90]*$'
(/likely/path being first the place where you launched nano from, then other such "possible" places, then in last resort: /
(of course, launch that last find command as root or expect a lot of error output, which you could redirect away using your shell's STDERR redirection)
-mtime -1 says "up to 1 day old", you may want to change the value to -2, or -3, depending on when you edited the file and when you read this.
In the event nano did not yet write such a file, you could try to send it a SIGHUP signal to force it to do so (see: http://en.wikipedia.org/wiki/Unix_signal#POSIX_signals )
And then, run the find again to look for that file...
And in last, last resort, you could play with grepping through /proc/kmem for parts of the text you are looking for, but this will necessitate some precautions to sanitize what it shows you, and could be not trivial. or dd it first into a (as big as your memory) file.
Solution 2
It does work as mentioned by @Oliver Dulac, but in some situations instead dumping the buffer to a file, nano just interpret and keep waiting from a user command.
There are more options without reboot:
pkill -SIGHUP -e nano
pkill -SIGTERM -e nano
pkill -SIGILL -e nano
but the program can choose to ignore those 3 signals above, hence try they in the order above, then check if the file was created, and if it do not work, try SIGKILL (that is sent after SIGTERM):
pkill -SIGKILL -e nano
keep in mind that SIGHUP, SIGTERM, and SIGILL can be caught or ignored by the running program, SIGKILL cannot.
- SIGHUP tell the program that the terminal in which it was running is closed, very common thing via remote commands.
- SIGTERM is the user signal to nicely close a program, but it can be ignored if the program needs something (i.e. should it save the buffer over the file?)
- SIGILL inform the program that it is performing something improper and should be closed, but it is still able to interpret and ignore as above;
- SIGKILL will cause the program to be immediately shut down, without it being able to react.
Solution 3
So, this may not be an option for you, but I just sent a reboot command to the box. When it came back online, Viola- file.py.save was put in the directory.
Related videos on Youtube
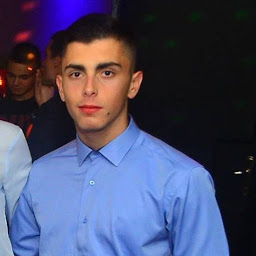
Yo yo
Updated on September 18, 2022Comments
-
Yo yo over 1 year
I'm coding JS app which will do this things
Find my location(I did this) Find specified ATM(well I didn't figure how to find around my radius) To list all found ATMs and sort them by distance
For this I need rankBy but I don't know how to use it.I looked on internet,where nearly everyone talks about that but no one don't show how to use.
Does someone can help mi to set my radius and in that radius to show ATMs and sort them by distance?
here is code
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>Marker Animations</title> <style> /* Always set the map height explicitly to define the size of the div * element that contains the map. */ #map { height: 100%; } /* Optional: Makes the sample page fill the window. */ html, body { height: 100%; margin: 0; padding: 0; } </style> </head> <body> <div id="map"></div> <script> function initMap() { var map, infoWindow,service,infoWindow1; map = new google.maps.Map(document.getElementById('map'), { center: {lat: -34.397, lng: 150.644}, zoom: 12 }); infoWindow = new google.maps.InfoWindow; // Try HTML5 geolocation. if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(function (position) { var pos = { lat: position.coords.latitude, lng: position.coords.longitude }; infoWindow.open(map); map.setCenter(pos); var marker = new google.maps.Marker({ map: map, position: pos, draggable: true, animation: google.maps.Animation.DROP, }); marker.addListener('click', toggleBounce); function toggleBounce() { if (marker.getAnimation() !== null) { marker.setAnimation(null); } else { marker.setAnimation(google.maps.Animation.BOUNCE); } } }, function () { handleLocationError(true, infoWindow, map.getCenter()); }); } else { // Browser doesn't support Geolocation handleLocationError(false, infoWindow, map.getCenter()); } function handleLocationError(browserHasGeolocation, infoWindow, pos) { infoWindow.setPosition(pos); infoWindow.setContent(browserHasGeolocation ? 'Error: The Geolocation service failed.' : 'Error: Your browser doesn\'t support geolocation.'); infoWindow.open(map); } infoWindow1 = new google.maps.InfoWindow; service = new google.maps.places.PlacesService(map); map.addListener('idle', performSearch); function performSearch() { var request = { bounds: map.getBounds(), keyword: 'telenor atm', }; service.radarSearch(request, callback); } function callback(results, status) { if (status !== google.maps.places.PlacesServiceStatus.OK) { console.error(status); return; } for (var i = 0, result; result = results[i]; i++) { addMarker(result); } } function addMarker(place) { var marker = new google.maps.Marker({ map: map, position: place.geometry.location, icon: { url: 'https://developers.google.com/maps/documentation/javascript/images/circle.png', anchor: new google.maps.Point(10, 10), scaledSize: new google.maps.Size(30, 37) } }); google.maps.event.addListener(marker, 'click', function () { service.getDetails(place, function (result, status) { if (status !== google.maps.places.PlacesServiceStatus.OK) { console.error(status); return; } infoWindow1.setContent(result.name); infoWindow1.open(map,marker); }); }); } } </script> <script src="https://maps.googleapis.com/maps/api/js?key=AIzaSyCW8gRR1ITJDx4F-rVpkBSetftu32XO2P0&callback=initMap&libraries=places,visualization" async defer></script> </body> </html>
-
Hecter over 11 yearsIncidentally, this question is a fantastic argument in favor of incorporating
screen
ortmux
into your daily workflow. If you'd been usingscreen
, then it would have been as simple asscreen -R
at login.
-
-
cusco over 6 yearsSending a HUP did not make it dump the .save file. However the reboot, like stated by @kevin-rutan bellow, worked!
-
pgr over 6 yearsGenius, this just saved my S
-
Tobias Beuving about 5 yearsHaleluja! Simple but spot on. In my case nano created a .swp file - tried opening and recovering it in vim - didn't work. Now with Kevins solution - when the system shuts down, nano creates a copy of the file that it has openend, and saves it to disk with .save appended to the filename. I was wrting a huge batch file, which I normally never do, so didn't exercise my save routine...
-
Jim over 4 yearsA complete reboot not needed. Just kill the process.
ps aux | grep nano
to get the PID, thenkill <PID>
it should create the *.save file for you. That way you don't interrupt any other services that may be running. -
Mark Booth about 3 yearsThe answer by Thiago Conrado details the steps to take if SIGHUP doesn't work.
-
Mark Booth about 3 yearsThis is a rather drastic solution, the answer by Thiago Conrado details the steps to take if SIGHUP doesn't work.
-
Admin about 2 years@kevin-rutan & @jim thanks a lot! I was testing Midnight Commander which uses Ctrl+O key binding which clashes with nano save file keybinding. As I was trying to save my nano document, MC stole the shortcut and crashed the nano session. But the PID was still running in the same terminal. I just did not see the contents. There was no .save file, only .swp, but that did not help. So I did what you suggested
killl <PID>
and the .save file was created. Perfect, thx again.